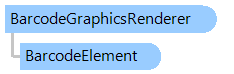
'Declaration Public MustInherit Class BarcodeGraphicsRenderer Inherits Vintasoft.Barcode.BarcodeRenderer
public abstract class BarcodeGraphicsRenderer : Vintasoft.Barcode.BarcodeRenderer
public __gc abstract class BarcodeGraphicsRenderer : public Vintasoft.Barcode.BarcodeRenderer*
public ref class BarcodeGraphicsRenderer abstract : public Vintasoft.Barcode.BarcodeRenderer^
This C#/VB.NET code shows how to create barcode renderer that overrides standard rendering algorithms.
Imports System.ComponentModel Imports System.Drawing Imports System.Drawing.Drawing2D Imports Vintasoft.Barcode Imports Vintasoft.Barcode.Gdi Imports Vintasoft.Barcode.BarcodeStructure Class BarcodeGraphicsRendererExample ''' <summary> ''' Generate QR barcode as an image. ''' </summary> ''' <param name="value">The value.</param> ''' <param name="width">The image width, in pixels.</param> ''' <param name="height">The image height, in pixels.</param> Public Shared Function GenerateQRBarcodeImage(value As String, width As Integer, height As Integer) As Bitmap Using writer As New BarcodeWriter() writer.Settings.Barcode = BarcodeType.QR writer.Settings.Value = value writer.Settings.Resolution = 96F writer.Settings.Padding = 0 Dim quietZone As Integer = Math.Min(width, height) \ 20 writer.Settings.QuietZoneTop = quietZone writer.Settings.QuietZoneLeft = quietZone writer.Settings.QuietZoneRight = quietZone writer.Settings.QuietZoneBottom = quietZone Dim renderer As New BarcodeSimpleRenderer() Return renderer.GetBarcodeAsBitmap(writer, width, height, UnitOfMeasure.Pixels) End Using End Function ''' <summary> ''' A simple renderer of matrix barcode. ''' </summary> ''' <remarks> ''' This renderer draws the barcode search patterns and other barcode elements separately. ''' </remarks> Public Class BarcodeSimpleRenderer Inherits BarcodeGraphicsRenderer #Region "Fields" ''' <summary> ''' The brush that is used for filling the barcode elements. ''' </summary> Private _barcodeBrush As New SolidBrush(Color.Black) ''' <summary> ''' The pen that is used for drawing the barcode elements. ''' </summary> Private _barcodePen As New Pen(Color.Black, 1) #End Region #Region "Constructors" ''' <summary> ''' Initializes a new instance of the <see cref="BarcodeSimpleRenderer"/> class. ''' </summary> Public Sub New() _barcodePen.LineJoin = LineJoin.Round End Sub #End Region #Region "Properties" ''' <summary> ''' Gets or sets the color that is used for drawing the barcode. ''' </summary> <Category("Colors")> _ <Description("Color that is used for drawing the barcode.")> _ Public Property BarcodeColor() As Color Get Return _barcodeBrush.Color End Get Set _barcodeBrush.Color = value _barcodePen.Color = value End Set End Property #End Region #Region "Methods" ''' <summary> ''' Renders the barcode matrix element. ''' </summary> ''' <param name="element">The barcode matrix element to render.</param> ''' <param name="x">The X-coordinate, where barcode matrix element must be rendered.</param> ''' <param name="y">The Y-coordinate, where barcode matrix element must be rendered.</param> Protected Overrides Sub RenderBarcodeMatrixElement(element As BarcodeMatrixElement, x As Integer, y As Integer) ' customize drawing of search patterns Dim isAztecSearchPattern As Boolean = False If element Is BarcodeElements.AztecCompactSearchPattern Then isAztecSearchPattern = True ElseIf element Is BarcodeElements.AztecFullRangeSearchPattern Then isAztecSearchPattern = True End If ' QR search pattern If element Is BarcodeElements.QrSearchPattern Then ' (x,y) ' ####### ' # # ' # ### # ' # ### # ' # ### # ' # # ' ####### ' (x+7,y+7) ' draw outer rectangle 7x7 Graphics.DrawRectangle(_barcodePen, x + 1.5F, y + 1.5F, 6, 6) ' draw inner cell 3x3 Graphics.FillRectangle(_barcodeBrush, x + 3.75F, y + 3.75F, 1.5F, 1.5F) Graphics.DrawRectangle(_barcodePen, x + 3.5F, y + 3.5F, 2F, 2F) ' Aztec search pattern ElseIf isAztecSearchPattern Then ' Compact/Rune Full Range ' (x,y) ' ############# ' (x,y) # # ' ######### # ######### # ' # # # # # # ' # ##### # # # ##### # # ' # # # # # # # # # # ' # # # # # # # # # # # # ' # # # # # # # # # # ' # ##### # # # ##### # # ' # # # # # # ' ######### # ######### # ' (x+9,y+9) # # ' ############# ' (x+13,y+13) ' draw outer rectangles: 13x13, 9x9, 5x5 Dim rectCount As Integer If element Is BarcodeElements.AztecFullRangeSearchPattern Then rectCount = 3 Else rectCount = 2 End If For i As Integer = 0 To rectCount - 1 Graphics.DrawRectangle(_barcodePen, x + 0.5F, y + 0.5F, (rectCount - i) * 4, (rectCount - i) * 4) x += 2 y += 2 Next ' draw inner cell 1x1 Graphics.FillEllipse(_barcodeBrush, New Rectangle(x, y, 1, 1)) ' DataMatrix and Han Xin Code search patterns ElseIf element.Name.Contains("Search Pattern") Then ' draw as single pattern use graphics path Using path As New GraphicsPath() For yc As Integer = 0 To element.Height - 1 For xc As Integer = 0 To element.Width - 1 If element.Matrix(yc, xc) Is BarcodeElements.BlackCell Then path.AddRectangle(New Rectangle(x + xc, y + yc, 1, 1)) End If Next Next Graphics.FillPath(_barcodeBrush, path) End Using Else MyBase.RenderBarcodeMatrixElement(element, x, y) End If End Sub ''' <summary> ''' Renders the barcode element. ''' </summary> ''' <param name="barcodeElement">The barcode element to render.</param> ''' <param name="x">The X-coordinate, where barcode element must be rendered.</param> ''' <param name="y">The Y-coordinate, where barcode element must be rendered.</param> Protected Overrides Sub RenderBarcodeElement(barcodeElement As BarcodeElement, x As Integer, y As Integer) If barcodeElement Is BarcodeElements.BlackCell Then Graphics.FillEllipse(_barcodeBrush, New Rectangle(x, y, 1, 1)) Else MyBase.RenderBarcodeElement(barcodeElement, x, y) End If End Sub ''' <summary> ''' Returns a <see cref="System.String" /> that represents this instance. ''' </summary> ''' <returns> ''' A <see cref="System.String" /> that represents this instance. ''' </returns> Public Overrides Function ToString() As String Return "Simple dots" End Function #End Region End Class End Class
using System; using System.ComponentModel; using System.Drawing; using System.Drawing.Drawing2D; using Vintasoft.Barcode; using Vintasoft.Barcode.Gdi; using Vintasoft.Barcode.BarcodeStructure; class BarcodeGraphicsRendererExample { /// <summary> /// Generate QR barcode as an image. /// </summary> /// <param name="value">The value.</param> /// <param name="width">The image width, in pixels.</param> /// <param name="height">The image height, in pixels.</param> public static Bitmap GenerateQRBarcodeImage(string value, int width, int height) { using (BarcodeWriter writer = new BarcodeWriter()) { writer.Settings.Barcode = BarcodeType.QR; writer.Settings.Value = value; writer.Settings.Resolution = 96f; writer.Settings.Padding = 0; int quietZone = Math.Min(width, height) / 20; writer.Settings.QuietZoneTop = quietZone; writer.Settings.QuietZoneLeft = quietZone; writer.Settings.QuietZoneRight = quietZone; writer.Settings.QuietZoneBottom = quietZone; BarcodeSimpleRenderer renderer = new BarcodeSimpleRenderer(); return renderer.GetBarcodeAsBitmap(writer, width, height, UnitOfMeasure.Pixels); } } /// <summary> /// A simple renderer of matrix barcode. /// </summary> /// <remarks> /// This renderer draws the barcode search patterns and other barcode elements separately. /// </remarks> public class BarcodeSimpleRenderer : BarcodeGraphicsRenderer { #region Fields /// <summary> /// The brush that is used for filling the barcode elements. /// </summary> SolidBrush _barcodeBrush = new SolidBrush(Color.Black); /// <summary> /// The pen that is used for drawing the barcode elements. /// </summary> Pen _barcodePen = new Pen(Color.Black, 1); #endregion #region Constructors /// <summary> /// Initializes a new instance of the <see cref="BarcodeSimpleRenderer"/> class. /// </summary> public BarcodeSimpleRenderer() { _barcodePen.LineJoin = LineJoin.Round; } #endregion #region Properties /// <summary> /// Gets or sets the color that is used for drawing the barcode. /// </summary> [Category("Colors")] [Description("Color that is used for drawing the barcode.")] public Color BarcodeColor { get { return _barcodeBrush.Color; } set { _barcodeBrush.Color = value; _barcodePen.Color = value; } } #endregion #region Methods /// <summary> /// Renders the barcode matrix element. /// </summary> /// <param name="element">The barcode matrix element to render.</param> /// <param name="x">The X-coordinate, where barcode matrix element must be rendered.</param> /// <param name="y">The Y-coordinate, where barcode matrix element must be rendered.</param> protected override void RenderBarcodeMatrixElement( BarcodeMatrixElement element, int x, int y) { // customize drawing of search patterns bool isAztecSearchPattern = false; if (element == BarcodeElements.AztecCompactSearchPattern) isAztecSearchPattern = true; else if (element == BarcodeElements.AztecFullRangeSearchPattern) isAztecSearchPattern = true; // QR search pattern if (element == BarcodeElements.QrSearchPattern) { // (x,y) // ####### // # # // # ### # // # ### # // # ### # // # # // ####### // (x+7,y+7) // draw outer rectangle 7x7 Graphics.DrawRectangle(_barcodePen, x + 1.5f, y + 1.5f, 6, 6); // draw inner cell 3x3 Graphics.FillRectangle(_barcodeBrush, x + 3.75f, y + 3.75f, 1.5f, 1.5f); Graphics.DrawRectangle(_barcodePen, x + 3.5f, y + 3.5f, 2f, 2f); } // Aztec search pattern else if (isAztecSearchPattern) { // Compact/Rune Full Range // (x,y) // ############# // (x,y) # # // ######### # ######### # // # # # # # # // # ##### # # # ##### # # // # # # # # # # # # # // # # # # # # # # # # # # // # # # # # # # # # # // # ##### # # # ##### # # // # # # # # # // ######### # ######### # // (x+9,y+9) # # // ############# // (x+13,y+13) // draw outer rectangles: 13x13, 9x9, 5x5 int rectCount; if (element == BarcodeElements.AztecFullRangeSearchPattern) rectCount = 3; else rectCount = 2; for (int i = 0; i < rectCount; i++) { Graphics.DrawRectangle(_barcodePen, x + 0.5f, y + 0.5f, (rectCount - i) * 4, (rectCount - i) * 4); x += 2; y += 2; } // draw inner cell 1x1 Graphics.FillEllipse(_barcodeBrush, new Rectangle(x, y, 1, 1)); } // DataMatrix and Han Xin Code search patterns else if (element.Name.Contains("Search Pattern")) { // draw as single pattern use graphics path using (GraphicsPath path = new GraphicsPath()) { for (int yc = 0; yc < element.Height; yc++) { for (int xc = 0; xc < element.Width; xc++) { if (element.Matrix[yc, xc] == BarcodeElements.BlackCell) path.AddRectangle(new Rectangle(x + xc, y + yc, 1, 1)); } } Graphics.FillPath(_barcodeBrush, path); } } else { base.RenderBarcodeMatrixElement(element, x, y); } } /// <summary> /// Renders the barcode element. /// </summary> /// <param name="barcodeElement">The barcode element to render.</param> /// <param name="x">The X-coordinate, where barcode element must be rendered.</param> /// <param name="y">The Y-coordinate, where barcode element must be rendered.</param> protected override void RenderBarcodeElement(BarcodeElement barcodeElement, int x, int y) { if (barcodeElement == BarcodeElements.BlackCell) { Graphics.FillEllipse(_barcodeBrush, new Rectangle(x, y, 1, 1)); } else { base.RenderBarcodeElement(barcodeElement, x, y); } } /// <summary> /// Returns a <see cref="System.String" /> that represents this instance. /// </summary> /// <returns> /// A <see cref="System.String" /> that represents this instance. /// </returns> public override string ToString() { return "Simple dots"; } #endregion } }
System.Object
 Vintasoft.Barcode.BarcodeRenderer
   Vintasoft.Barcode.Gdi.BarcodeGraphicsRenderer
Target Platforms: .NET9; .NET 8; .NET 7; .NET 6; .NET Framework 4.8, 4.7, 4.6, 4.5, 4.0, 3.5