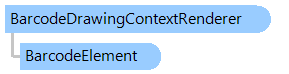
'Declaration Public MustInherit Class BarcodeDrawingContextRenderer Inherits Vintasoft.Barcode.BarcodeRenderer
public abstract class BarcodeDrawingContextRenderer : Vintasoft.Barcode.BarcodeRenderer
public __gc abstract class BarcodeDrawingContextRenderer : public Vintasoft.Barcode.BarcodeRenderer*
public ref class BarcodeDrawingContextRenderer abstract : public Vintasoft.Barcode.BarcodeRenderer^
This C#/VB.NET code shows how to create barcode renderer that overrides standard rendering algorithms.
''' <summary> ''' Generate QR barcode as an image. ''' </summary> ''' <param name="value">The value.</param> ''' <param name="width">The image width, in pixels.</param> ''' <param name="height">The image height, in pixels.</param> Public Shared Function GenerateQRBarcodeImage(value As String, width As Integer, height As Integer) As BitmapSource Dim writer As New BarcodeWriter() writer.Settings.Barcode = BarcodeType.QR writer.Settings.Value = value writer.Settings.Resolution = 96F writer.Settings.Padding = 0 Dim quietZone As Integer = Math.Min(width, height) \ 20 writer.Settings.QuietZoneTop = quietZone writer.Settings.QuietZoneLeft = quietZone writer.Settings.QuietZoneRight = quietZone writer.Settings.QuietZoneBottom = quietZone Dim renderer As New BarcodeSimpleRenderer() Return renderer.GetBarcodeAsBitmap(writer, width, height, UnitOfMeasure.Pixels) End Function ''' <summary> ''' A simple renderer of matrix barcode. ''' </summary> ''' <remarks> ''' This renderer draws the barcode search patterns and other barcode elements separately. ''' </remarks> Public Class BarcodeSimpleRenderer Inherits BarcodeDrawingContextRenderer #Region "Fields" ''' <summary> ''' The brush that is used for filling the barcode elements. ''' </summary> Private _barcodeBrush As SolidColorBrush ''' <summary> ''' The pen that is used for drawing the barcode elements. ''' </summary> Private _barcodePen As Pen ''' <summary> ''' The geometry that is used for drawing the barcode elements. ''' </summary> Private _drawGeometry As GeometryGroup ''' <summary> ''' The geometry that is used for filling the barcode elements. ''' </summary> Private _fillGeometry As GeometryGroup #End Region #Region "Constructors" ''' <summary> ''' Initializes a new instance of the <see cref="BarcodeSimpleRenderer"/> class. ''' </summary> Public Sub New() End Sub #End Region #Region "Properties" Private _barcodeColor As Color = Colors.Black ''' <summary> ''' Gets or sets the color that is used for drawing the barcode. ''' </summary> Public Property BarcodeColor() As Color Get Return _barcodeColor End Get Set _barcodeColor = value End Set End Property #End Region #Region "Methods" ''' <summary> ''' Renders the barcode. ''' </summary> ''' <seealso cref="BarcodeElement" /> Public Overrides Sub Render() ' clear groups _fillGeometry = New GeometryGroup() _drawGeometry = New GeometryGroup() MyBase.Render() ' create brush _barcodeBrush = New SolidColorBrush(BarcodeColor) _barcodeBrush.Freeze() ' create pen _barcodePen = New Pen(_barcodeBrush, 1) _barcodePen.LineJoin = PenLineJoin.Round _barcodePen.Freeze() ' draw groups _fillGeometry.Freeze() _drawGeometry.Freeze() DrawingContext.DrawGeometry(_barcodeBrush, Nothing, _fillGeometry) DrawingContext.DrawGeometry(Nothing, _barcodePen, _drawGeometry) End Sub ''' <summary> ''' Renders the barcode matrix element. ''' </summary> ''' <param name="element">The barcode matrix element to render.</param> ''' <param name="x">The X-coordinate, where barcode matrix element must be rendered.</param> ''' <param name="y">The Y-coordinate, where barcode matrix element must be rendered.</param> Protected Overrides Sub RenderBarcodeMatrixElement(element As BarcodeMatrixElement, x As Integer, y As Integer) ' customize drawing of search patterns Dim isAztecSearchPattern As Boolean = False If element Is BarcodeElements.AztecCompactSearchPattern Then isAztecSearchPattern = True ElseIf element Is BarcodeElements.AztecFullRangeSearchPattern Then isAztecSearchPattern = True End If ' QR search pattern If element Is BarcodeElements.QrSearchPattern Then ' (x,y) ' ####### ' # # ' # ### # ' # ### # ' # ### # ' # # ' ####### ' (x+7,y+7) ' draw outer rectangle 7x7 _drawGeometry.Children.Add(New RectangleGeometry(New Rect(x + 1.5, y + 1.5, 6, 6))) ' draw inner cell 3x3 _fillGeometry.Children.Add(New RectangleGeometry(New Rect(x + 3.75, y + 3.75, 1.5, 1.5))) _drawGeometry.Children.Add(New RectangleGeometry(New Rect(x + 3.5, y + 3.5, 2, 2))) ' Aztec search pattern ElseIf isAztecSearchPattern Then ' Compact/Rune Full Range ' (x,y) ' ############# ' (x,y) # # ' ######### # ######### # ' # # # # # # ' # ##### # # # ##### # # ' # # # # # # # # # # ' # # # # # # # # # # # # ' # # # # # # # # # # ' # ##### # # # ##### # # ' # # # # # # ' ######### # ######### # ' (x+9,y+9) # # ' ############# ' (x+13,y+13) ' draw outer rectangles: 13x13, 9x9, 5x5 Dim rectCount As Integer If element Is BarcodeElements.AztecFullRangeSearchPattern Then rectCount = 3 Else rectCount = 2 End If For i As Integer = 0 To rectCount - 1 _drawGeometry.Children.Add(New RectangleGeometry(New Rect(x + 0.5, y + 0.5, (rectCount - i) * 4, (rectCount - i) * 4))) x += 2 y += 2 Next ' draw inner cell 1x1 Dim center As New Point(x + 0.5, y + 0.5) _fillGeometry.Children.Add(New EllipseGeometry(center, 0.5, 0.5)) ' DataMatrix and Han Xin Code search patterns ElseIf element.Name.Contains("Search Pattern") Then For yc As Integer = 0 To element.Height - 1 For xc As Integer = 0 To element.Width - 1 If element.Matrix(yc, xc) Is BarcodeElements.BlackCell Then Dim rect As New Rect(x + xc, y + yc, 1, 1) Dim rectangle As New RectangleGeometry(rect) _fillGeometry.Children.Add(rectangle) End If Next Next Else MyBase.RenderBarcodeMatrixElement(element, x, y) End If End Sub ''' <summary> ''' Renders the barcode element. ''' </summary> ''' <param name="barcodeElement">The barcode element to render.</param> ''' <param name="x">The X-coordinate, where barcode element must be rendered.</param> ''' <param name="y">The Y-coordinate, where barcode element must be rendered.</param> Protected Overrides Sub RenderBarcodeElement(barcodeElement As BarcodeElement, x As Integer, y As Integer) If barcodeElement Is BarcodeElements.BlackCell Then Dim center As New Point(x + 0.5, y + 0.5) _fillGeometry.Children.Add(New EllipseGeometry(center, 0.5, 0.5)) Else MyBase.RenderBarcodeElement(barcodeElement, x, y) End If End Sub ''' <summary> ''' Returns a <see cref="System.String" /> that represents this instance. ''' </summary> ''' <returns> ''' A <see cref="System.String" /> that represents this instance. ''' </returns> Public Overrides Function ToString() As String Return "Simple dots" End Function #End Region End Class
/// <summary> /// Generate QR barcode as an image. /// </summary> /// <param name="value">The value.</param> /// <param name="width">The image width, in pixels.</param> /// <param name="height">The image height, in pixels.</param> public static BitmapSource GenerateQRBarcodeImage(string value, int width, int height) { BarcodeWriter writer = new BarcodeWriter(); writer.Settings.Barcode = BarcodeType.QR; writer.Settings.Value = value; writer.Settings.Resolution = 96f; writer.Settings.Padding = 0; int quietZone = Math.Min(width, height) / 20; writer.Settings.QuietZoneTop = quietZone; writer.Settings.QuietZoneLeft = quietZone; writer.Settings.QuietZoneRight = quietZone; writer.Settings.QuietZoneBottom = quietZone; BarcodeSimpleRenderer renderer = new BarcodeSimpleRenderer(); return renderer.GetBarcodeAsBitmap(writer, width, height, UnitOfMeasure.Pixels); } /// <summary> /// A simple renderer of matrix barcode. /// </summary> /// <remarks> /// This renderer draws the barcode search patterns and other barcode elements separately. /// </remarks> public class BarcodeSimpleRenderer : BarcodeDrawingContextRenderer { #region Fields /// <summary> /// The brush that is used for filling the barcode elements. /// </summary> SolidColorBrush _barcodeBrush; /// <summary> /// The pen that is used for drawing the barcode elements. /// </summary> Pen _barcodePen; /// <summary> /// The geometry that is used for drawing the barcode elements. /// </summary> GeometryGroup _drawGeometry; /// <summary> /// The geometry that is used for filling the barcode elements. /// </summary> GeometryGroup _fillGeometry; #endregion #region Constructors /// <summary> /// Initializes a new instance of the <see cref="BarcodeSimpleRenderer"/> class. /// </summary> public BarcodeSimpleRenderer() { } #endregion #region Properties Color _barcodeColor = Colors.Black; /// <summary> /// Gets or sets the color that is used for drawing the barcode. /// </summary> public Color BarcodeColor { get { return _barcodeColor; } set { _barcodeColor = value; } } #endregion #region Methods /// <summary> /// Renders the barcode. /// </summary> /// <seealso cref="BarcodeElement" /> public override void Render() { // clear groups _fillGeometry = new GeometryGroup(); _drawGeometry = new GeometryGroup(); base.Render(); // create brush _barcodeBrush = new SolidColorBrush(BarcodeColor); _barcodeBrush.Freeze(); // create pen _barcodePen = new Pen(_barcodeBrush, 1); _barcodePen.LineJoin = PenLineJoin.Round; _barcodePen.Freeze(); // draw groups _fillGeometry.Freeze(); _drawGeometry.Freeze(); DrawingContext.DrawGeometry(_barcodeBrush, null, _fillGeometry); DrawingContext.DrawGeometry(null, _barcodePen, _drawGeometry); } /// <summary> /// Renders the barcode matrix element. /// </summary> /// <param name="element">The barcode matrix element to render.</param> /// <param name="x">The X-coordinate, where barcode matrix element must be rendered.</param> /// <param name="y">The Y-coordinate, where barcode matrix element must be rendered.</param> protected override void RenderBarcodeMatrixElement( BarcodeMatrixElement element, int x, int y) { // customize drawing of search patterns bool isAztecSearchPattern = false; if (element == BarcodeElements.AztecCompactSearchPattern) isAztecSearchPattern = true; else if (element == BarcodeElements.AztecFullRangeSearchPattern) isAztecSearchPattern = true; // QR search pattern if (element == BarcodeElements.QrSearchPattern) { // (x,y) // ####### // # # // # ### # // # ### # // # ### # // # # // ####### // (x+7,y+7) // draw outer rectangle 7x7 _drawGeometry.Children.Add(new RectangleGeometry(new Rect(x + 1.5, y + 1.5, 6, 6))); // draw inner cell 3x3 _fillGeometry.Children.Add(new RectangleGeometry(new Rect(x + 3.75, y + 3.75, 1.5, 1.5))); _drawGeometry.Children.Add(new RectangleGeometry(new Rect(x + 3.5, y + 3.5, 2, 2))); } // Aztec search pattern else if (isAztecSearchPattern) { // Compact/Rune Full Range // (x,y) // ############# // (x,y) # # // ######### # ######### # // # # # # # # // # ##### # # # ##### # # // # # # # # # # # # # // # # # # # # # # # # # # // # # # # # # # # # # // # ##### # # # ##### # # // # # # # # # // ######### # ######### # // (x+9,y+9) # # // ############# // (x+13,y+13) // draw outer rectangles: 13x13, 9x9, 5x5 int rectCount; if (element == BarcodeElements.AztecFullRangeSearchPattern) rectCount = 3; else rectCount = 2; for (int i = 0; i < rectCount; i++) { _drawGeometry.Children.Add(new RectangleGeometry( new Rect(x + 0.5, y + 0.5, (rectCount - i) * 4, (rectCount - i) * 4))); x += 2; y += 2; } // draw inner cell 1x1 Point center = new Point(x + 0.5, y + 0.5); _fillGeometry.Children.Add(new EllipseGeometry(center, 0.5, 0.5)); } // DataMatrix and Han Xin Code search patterns else if (element.Name.Contains("Search Pattern")) { for (int yc = 0; yc < element.Height; yc++) { for (int xc = 0; xc < element.Width; xc++) { if (element.Matrix[yc, xc] == BarcodeElements.BlackCell) { Rect rect = new Rect(x + xc, y + yc, 1, 1); RectangleGeometry rectangle = new RectangleGeometry(rect); _fillGeometry.Children.Add(rectangle); } } } } else { base.RenderBarcodeMatrixElement(element, x, y); } } /// <summary> /// Renders the barcode element. /// </summary> /// <param name="barcodeElement">The barcode element to render.</param> /// <param name="x">The X-coordinate, where barcode element must be rendered.</param> /// <param name="y">The Y-coordinate, where barcode element must be rendered.</param> protected override void RenderBarcodeElement(BarcodeElement barcodeElement, int x, int y) { if (barcodeElement == BarcodeElements.BlackCell) { Point center = new Point(x + 0.5, y + 0.5); _fillGeometry.Children.Add(new EllipseGeometry(center, 0.5, 0.5)); } else { base.RenderBarcodeElement(barcodeElement, x, y); } } /// <summary> /// Returns a <see cref="System.String" /> that represents this instance. /// </summary> /// <returns> /// A <see cref="System.String" /> that represents this instance. /// </returns> public override string ToString() { return "Simple dots"; } #endregion }
System.Object
 Vintasoft.Barcode.BarcodeRenderer
   Vintasoft.Barcode.Wpf.BarcodeDrawingContextRenderer
Target Platforms: .NET9; .NET 8; .NET 7; .NET 6; .NET Framework 4.8, 4.7, 4.6, 4.5, 4.0, 3.5
BarcodeDrawingContextRenderer Members
Vintasoft.Barcode.Wpf Namespace
MatrixBarcodeStructure