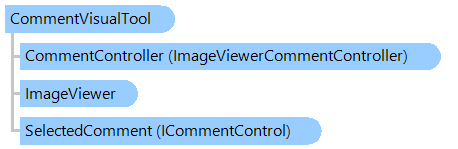
public class CommentVisualTool : Vintasoft.Imaging.UI.VisualTools.VisualTool
public __gc class CommentVisualTool : public Vintasoft.Imaging.UI.VisualTools.VisualTool*
public ref class CommentVisualTool : public Vintasoft.Imaging.UI.VisualTools.VisualTool^
'Declaration Public Class CommentVisualTool Inherits Vintasoft.Imaging.UI.VisualTools.VisualTool
This C#/VB.NET code shows how to initialize WinForms annotation viewer for displaying the annotation comments.
''' <summary> ''' Initializes WinForms image viewer for displaying PDF annotation comments. ''' </summary> ''' <param name="imageViewer">WinForms image viewer, which must be initialized for displaying PDF annotation comments.</param> Public Shared Sub InitViewerToDisplayPdfAnnotationComments(imageViewer As Vintasoft.Imaging.UI.ImageViewer) ' create new PDF annotation comment controller Dim annotationCommentController As New Vintasoft.Imaging.Annotation.Comments.Pdf.ImageCollectionPdfAnnotationCommentController() ' create the comment visual tool, which is associated with PDF annotation comment controller Dim commentVisualTool As Vintasoft.Imaging.Annotation.UI.VisualTools.CommentVisualTool = CreateCommentTool(annotationCommentController) ' specify that comment visual tool must be used in WinForms image viewer imageViewer.VisualTool = New Vintasoft.Imaging.UI.VisualTools.CompositeVisualTool(commentVisualTool, imageViewer.VisualTool) End Sub ''' <summary> ''' Initializes WinForms annotation viewer for displaying Vintasoft annotation comments. ''' </summary> ''' <param name="annotationViewer">WinForms annotation viewer, which must be initialized for displaying Vintasoft annotation comments.</param> Public Shared Sub InitViewerToDisplayVintasoftAnnotationComments(annotationViewer As Vintasoft.Imaging.Annotation.UI.AnnotationViewer) ' create the annotation comment controller, which is associated with annotation data controller Dim annotationCommentController As New Vintasoft.Imaging.Annotation.Comments.AnnotationCommentController(annotationViewer.AnnotationDataController) ' create the comment visual tool, which is associated with annotation comment controller Dim commentVisualTool As Vintasoft.Imaging.Annotation.UI.VisualTools.CommentVisualTool = CreateCommentTool(annotationCommentController) ' specify that comment visual tool must be used in WinForms annotation viewer annotationViewer.VisualTool = New Vintasoft.Imaging.UI.VisualTools.CompositeVisualTool(commentVisualTool, annotationViewer.VisualTool) End Sub ''' <summary> ''' Creates the comment visual tool using the specified comment controller. ''' </summary> ''' <param name="imageCollectionCommentController">The image collection comment controller.</param> ''' <returns>The comment visual tool.</returns> Public Shared Function CreateCommentTool(imageCollectionCommentController As Vintasoft.Imaging.Annotation.Comments.ImageCollectionCommentController) As Vintasoft.Imaging.Annotation.UI.VisualTools.CommentVisualTool ' create the comment controller for WinForms image viewer Dim imageViewerCommentController As New Vintasoft.Imaging.Annotation.UI.Comments.ImageViewerCommentController(imageCollectionCommentController) ' create the comment visual tool, which uses the CommentControlFactory class for creating the comment controls Return New Vintasoft.Imaging.Annotation.UI.VisualTools.CommentVisualTool(imageViewerCommentController, New CommentControlFactory()) End Function ''' <summary> ''' Represents a factory that allows to create comment controls. ''' </summary> Public Class CommentControlFactory Implements Vintasoft.Imaging.Annotation.UI.Comments.ICommentControlFactory ''' <summary> ''' Creates the comment control that displays specified comment in WinForms image viewer. ''' </summary> ''' <param name="comment">The comment.</param> ''' <returns>A comment control that displays comment in WinForms image viewer.</returns> Public Function Create(comment As Vintasoft.Imaging.Annotation.Comments.Comment) As Vintasoft.Imaging.Annotation.UI.Comments.ICommentControl Implements Vintasoft.Imaging.Annotation.UI.Comments.ICommentControlFactory.Create ' create a comment control that displays comment in WinForms image viewer Return New CommentControl(comment) End Function End Class ' see code of CommentControl class for PDF annotation comment in file ' "%INSTALL_PATH%\Examples\WinForms\CSharp\PdfEditorDemo\DemosCommonCode.Annotation\Comments\CommentControl.cs" ' ' see code of CommentControl class for Vintasoft annotation comment in file ' "%INSTALL_PATH%\Examples\WinForms\CSharp\AnnotationDemo\DemosCommonCode.Annotation\Comments\CommentControl.cs"
/// <summary> /// Initializes WinForms image viewer for displaying PDF annotation comments. /// </summary> /// <param name="imageViewer">WinForms image viewer, which must be initialized for displaying PDF annotation comments.</param> public static void InitViewerToDisplayPdfAnnotationComments(Vintasoft.Imaging.UI.ImageViewer imageViewer) { // create new PDF annotation comment controller Vintasoft.Imaging.Annotation.Comments.Pdf.ImageCollectionPdfAnnotationCommentController annotationCommentController = new Vintasoft.Imaging.Annotation.Comments.Pdf.ImageCollectionPdfAnnotationCommentController(); // create the comment visual tool, which is associated with PDF annotation comment controller Vintasoft.Imaging.Annotation.UI.VisualTools.CommentVisualTool commentVisualTool = CreateCommentTool(annotationCommentController); // specify that comment visual tool must be used in WinForms image viewer imageViewer.VisualTool = new Vintasoft.Imaging.UI.VisualTools.CompositeVisualTool(commentVisualTool, imageViewer.VisualTool); } /// <summary> /// Initializes WinForms annotation viewer for displaying Vintasoft annotation comments. /// </summary> /// <param name="annotationViewer">WinForms annotation viewer, which must be initialized for displaying Vintasoft annotation comments.</param> public static void InitViewerToDisplayVintasoftAnnotationComments(Vintasoft.Imaging.Annotation.UI.AnnotationViewer annotationViewer) { // create the annotation comment controller, which is associated with annotation data controller Vintasoft.Imaging.Annotation.Comments.AnnotationCommentController annotationCommentController = new Vintasoft.Imaging.Annotation.Comments.AnnotationCommentController(annotationViewer.AnnotationDataController); // create the comment visual tool, which is associated with annotation comment controller Vintasoft.Imaging.Annotation.UI.VisualTools.CommentVisualTool commentVisualTool = CreateCommentTool(annotationCommentController); // specify that comment visual tool must be used in WinForms annotation viewer annotationViewer.VisualTool = new Vintasoft.Imaging.UI.VisualTools.CompositeVisualTool(commentVisualTool, annotationViewer.VisualTool); } /// <summary> /// Creates the comment visual tool using the specified comment controller. /// </summary> /// <param name="imageCollectionCommentController">The image collection comment controller.</param> /// <returns>The comment visual tool.</returns> public static Vintasoft.Imaging.Annotation.UI.VisualTools.CommentVisualTool CreateCommentTool( Vintasoft.Imaging.Annotation.Comments.ImageCollectionCommentController imageCollectionCommentController) { // create the comment controller for WinForms image viewer Vintasoft.Imaging.Annotation.UI.Comments.ImageViewerCommentController imageViewerCommentController = new Vintasoft.Imaging.Annotation.UI.Comments.ImageViewerCommentController(imageCollectionCommentController); // create the comment visual tool, which uses the CommentControlFactory class for creating the comment controls return new Vintasoft.Imaging.Annotation.UI.VisualTools.CommentVisualTool(imageViewerCommentController, new CommentControlFactory()); } /// <summary> /// Represents a factory that allows to create comment controls. /// </summary> public class CommentControlFactory : Vintasoft.Imaging.Annotation.UI.Comments.ICommentControlFactory { /// <summary> /// Creates the comment control that displays specified comment in WinForms image viewer. /// </summary> /// <param name="comment">The comment.</param> /// <returns>A comment control that displays comment in WinForms image viewer.</returns> public Vintasoft.Imaging.Annotation.UI.Comments.ICommentControl Create(Vintasoft.Imaging.Annotation.Comments.Comment comment) { // create a comment control that displays comment in WinForms image viewer return new CommentControl(comment); } } // see code of CommentControl class for PDF annotation comment in file // "%INSTALL_PATH%\Examples\WinForms\CSharp\PdfEditorDemo\DemosCommonCode.Annotation\Comments\CommentControl.cs" // // see code of CommentControl class for Vintasoft annotation comment in file // "%INSTALL_PATH%\Examples\WinForms\CSharp\AnnotationDemo\DemosCommonCode.Annotation\Comments\CommentControl.cs"
System.Object
Vintasoft.Imaging.UI.VisualTools.VisualTool
Vintasoft.Imaging.Annotation.UI.VisualTools.CommentVisualTool
Target Platforms: .NET9; .NET 8; .NET 7; .NET 6; .NET Framework 4.8, 4.7, 4.6, 4.5, 4.0, 3.5