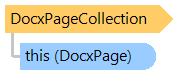
'Declaration <DefaultMemberAttribute("Item")> Public Class DocxPageCollection Inherits Vintasoft.Imaging.Office.OpenXml.OpenXmlPageCollection(Of TPage,TDocument)
[DefaultMember("Item")] public class DocxPageCollection : Vintasoft.Imaging.Office.OpenXml.OpenXmlPageCollection<TPage,TDocument>
[DefaultMember("Item")] public __gc class DocxPageCollection : public Vintasoft.Imaging.Office.OpenXml.OpenXmlPageCollection<TPage,TDocument*>*
[DefaultMember("Item")] public ref class DocxPageCollection : public Vintasoft.Imaging.Office.OpenXml.OpenXmlPageCollection<TPage,TDocument^>^
Here is an example that shows how to render all pages of DOCX document and save rendered images to PNG files:
System.Object
 Vintasoft.Imaging.Office.OpenXml.OpenXmlPageCollection<Vintasoft.Imaging.Office.OpenXml.Docx.DocxPage,Vintasoft.Imaging.Office.OpenXml.Docx.DocxDocument>
   Vintasoft.Imaging.Office.OpenXml.Docx.DocxPageCollection
Target Platforms: .NET9; .NET 8; .NET 7; .NET 6; .NET Framework 4.8, 4.7, 4.6, 4.5, 4.0, 3.5