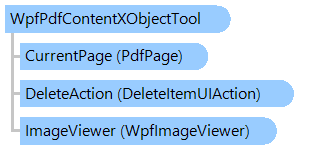
'Declaration <ToolboxItemAttribute("ToolboxItemType = null", "ToolboxItemTypeName = ")> <DesignTimeVisibleAttribute("Visible = False")> <DefaultPropertyAttribute("Content")> <ContentPropertyAttribute("Content")> <LocalizabilityAttribute(None)> <StyleTypedPropertyAttribute("Property = FocusVisualStyle", "StyleTargetType = System.Windows.Controls.Control")> <XmlLangPropertyAttribute("Name = Language")> <UsableDuringInitializationAttribute("Usable = True")> <RuntimeNamePropertyAttribute("Name = Name")> <UidPropertyAttribute()> <TypeDescriptionProviderAttribute("TypeName = MS.Internal.ComponentModel.DependencyObjectProvider")> <NameScopePropertyAttribute("Name = NameScope", "Type = System.Windows.NameScope")> Public Class WpfPdfContentXObjectTool Inherits WpfPdfVisualTool Implements ISupportUIActions
[ToolboxItem("ToolboxItemType = null", "ToolboxItemTypeName = ")] [DesignTimeVisible("Visible = False")] [DefaultProperty("Content")] [ContentProperty("Content")] [Localizability(None)] [StyleTypedProperty("Property = FocusVisualStyle", "StyleTargetType = System.Windows.Controls.Control")] [XmlLangProperty("Name = Language")] [UsableDuringInitialization("Usable = True")] [RuntimeNameProperty("Name = Name")] [UidProperty()] [TypeDescriptionProvider("TypeName = MS.Internal.ComponentModel.DependencyObjectProvider")] [NameScopeProperty("Name = NameScope", "Type = System.Windows.NameScope")] public class WpfPdfContentXObjectTool : WpfPdfVisualTool, ISupportUIActions
[ToolboxItem("ToolboxItemType = null", "ToolboxItemTypeName = ")] [DesignTimeVisible("Visible = False")] [DefaultProperty("Content")] [ContentProperty("Content")] [Localizability(None)] [StyleTypedProperty("Property = FocusVisualStyle", "StyleTargetType = System.Windows.Controls.Control")] [XmlLangProperty("Name = Language")] [UsableDuringInitialization("Usable = True")] [RuntimeNameProperty("Name = Name")] [UidProperty()] [TypeDescriptionProvider("TypeName = MS.Internal.ComponentModel.DependencyObjectProvider")] [NameScopeProperty("Name = NameScope", "Type = System.Windows.NameScope")] public __gc class WpfPdfContentXObjectTool : public WpfPdfVisualTool*, ISupportUIActions
[ToolboxItem("ToolboxItemType = null", "ToolboxItemTypeName = ")] [DesignTimeVisible("Visible = False")] [DefaultProperty("Content")] [ContentProperty("Content")] [Localizability(None)] [StyleTypedProperty("Property = FocusVisualStyle", "StyleTargetType = System.Windows.Controls.Control")] [XmlLangProperty("Name = Language")] [UsableDuringInitialization("Usable = True")] [RuntimeNameProperty("Name = Name")] [UidProperty()] [TypeDescriptionProvider("TypeName = MS.Internal.ComponentModel.DependencyObjectProvider")] [NameScopeProperty("Name = NameScope", "Type = System.Windows.NameScope")] public ref class WpfPdfContentXObjectTool : public WpfPdfVisualTool^, ISupportUIActions
This C#/VB.NET code shows how to highlight focused XObject in PDF document loaded into image viewer.
System.Object
 System.Windows.Threading.DispatcherObject
   System.Windows.DependencyObject
     System.Windows.Media.Visual
       System.Windows.UIElement
         System.Windows.FrameworkElement
           System.Windows.Controls.Control
             System.Windows.Controls.ContentControl
               Vintasoft.Imaging.Wpf.UI.VisualTools.WpfVisualTool
                 Vintasoft.Imaging.Pdf.Wpf.UI.WpfPdfVisualTool
                   Vintasoft.Imaging.Pdf.Wpf.UI.WpfPdfContentXObjectTool
Target Platforms: .NET9; .NET 8; .NET 7; .NET 6; .NET Framework 4.8, 4.7, 4.6, 4.5, 4.0, 3.5