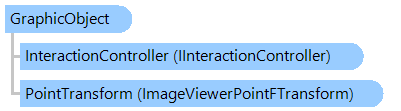
'Declaration Public MustInherit Class GraphicObject Implements IInteractiveObject
public abstract class GraphicObject : IInteractiveObject
public __gc abstract class GraphicObject : IInteractiveObject
public ref class GraphicObject abstract : IInteractiveObject
By default the coordinate space of object is equal to the coordinate space of image viewer, i.e. object point with coordinate (0;0) coincides with the left-top point of image viewer.
The coordinate space of object can be changed using the PointTransform property. Example that shows how to create static rectangle which has coordinates coincident with image coordinates can be found in Examples section.
This C#/VB.NET code shows how to create a grid over an image in image viewer:
''' <summary> ''' Displays grid over image in image viewer. ''' </summary> ''' <param name="viewer">The viewer.</param> Public Shared Sub DrawGridOverImageInImageViewer(viewer As Vintasoft.Imaging.UI.ImageViewer) ' create visual tool that can display static graphic objects in an image viewer Dim tool As New Vintasoft.Imaging.UI.VisualTools.GraphicObjects.GraphicObjectTool() ' create graphic object that displays image grid Dim grid As New GridGraphicObject(tool) ' add graphic object to the visual tool tool.GraphicObjectCollection.Add(grid) ' set visual tool as current visual tool of image viewer viewer.VisualTool = tool End Sub ''' <summary> ''' Represents an image grid which can be displayed in image viewer. ''' </summary> Public Class GridGraphicObject Inherits Vintasoft.Imaging.UI.VisualTools.GraphicObjects.GraphicObject #Region "Fields" Private _tool As Vintasoft.Imaging.UI.VisualTools.VisualTool #End Region #Region "Constructors" ''' <summary> ''' Initializes a new instance of the <see cref="GridGraphicObject"/> class. ''' </summary> ''' <param name="tool">The visual tool.</param> Public Sub New(tool As Vintasoft.Imaging.UI.VisualTools.VisualTool) If tool Is Nothing Then Throw New System.ArgumentNullException() End If _tool = tool Pen = New System.Drawing.Pen(System.Drawing.Color.FromArgb(128, System.Drawing.Color.Lime), 1) PointTransform = New Vintasoft.Imaging.UI.VisualTools.PixelsToImageViewerPointFTransform() End Sub #End Region #Region "Properties" Private _location As System.Drawing.PointF = System.Drawing.PointF.Empty ''' <summary> ''' Gets or sets the location of grid. ''' </summary> ''' <value> ''' Default value is <b>(0,0)</b>. ''' </value> Public Property Location() As System.Drawing.PointF Get Return _location End Get Set _location = value End Set End Property Private _cellSize As New System.Drawing.SizeF(1, 1) ''' <summary> ''' Gets or sets the size of the grid cell. ''' </summary> ''' <value> ''' Default value is <b>(1,1)</b>. ''' </value> Public Property CellSize() As System.Drawing.SizeF Get Return _cellSize End Get Set _cellSize = value End Set End Property Private _cellUnitOfMeasure As Vintasoft.Imaging.UnitOfMeasure = Vintasoft.Imaging.UnitOfMeasure.Centimeters ''' <summary> ''' Gets or sets the unit of measure of the grid cell. ''' </summary> ''' <value> ''' Default value is <b>UnitOfMeasure.Centimeters</b>. ''' </value> Public Property CellUnitOfMeasure() As Vintasoft.Imaging.UnitOfMeasure Get Return _cellUnitOfMeasure End Get Set _cellUnitOfMeasure = value End Set End Property #End Region #Region "Methods" ''' <summary> ''' Returns a value indicating whether point belongs the object. ''' </summary> ''' <param name="x">X coordinate of point in object space.</param> ''' <param name="y">Y coordinate of point in object space.</param> ''' <returns> ''' <b>true</b> if point belongs the object; ''' otherwise, <b>false</b>. ''' </returns> Public Overrides Function IsPointOnObject(x As Single, y As Single) As Boolean Return IsPointOnObject(x, y, False) End Function ''' <summary> ''' Returns a value indicating whether point belongs the object. ''' </summary> ''' <param name="x">X coordinate of point in object space.</param> ''' <param name="y">Y coordinate of point in object space.</param> ''' <param name="ignoreContainmentCheckDistance">A value indicating whether the point must be checked on the object only (ignore ''' the "containment" region around object that is used for object selection).</param> ''' <returns> ''' <b>true</b> if point belongs the object; ''' otherwise, <b>false</b>. ''' </returns> Public Overrides Function IsPointOnObject(x As Single, y As Single, ignoreContainmentCheckDistance As Boolean) As Boolean If _tool.ImageViewer.Image IsNot Nothing AndAlso (Pen IsNot Nothing OrElse Brush IsNot Nothing) Then Dim rect As System.Drawing.RectangleF = GetRectangle(_location, _tool.ImageViewer.Image) Return rect.Contains(x, y) Else Return False End If End Function ''' <summary> ''' Returns a bounding box of object, in object space. ''' </summary> ''' <returns> ''' Bounding box of object, in object space. ''' </returns> Public Overrides Function GetBoundingBox() As System.Drawing.RectangleF If _tool.ImageViewer.Image IsNot Nothing Then Return GetRectangle(_location, _tool.ImageViewer.Image) Else Return System.Drawing.RectangleF.Empty End If End Function ''' <summary> ''' Returns a drawing rectangle of object, in object space. ''' </summary> ''' <param name="viewer">An image viewer.</param> ''' <returns> ''' Drawing rectangle of object, in object space. ''' </returns> Public Overrides Function GetDrawingBox(viewer As Vintasoft.Imaging.UI.ImageViewer) As System.Drawing.RectangleF If viewer.Image Is Nothing Then Return System.Drawing.RectangleF.Empty End If Dim rect As System.Drawing.RectangleF = GetRectangle(_location, viewer.Image) If Pen IsNot Nothing Then Dim penWidth As Single = Pen.Width / 2F rect.Inflate(penWidth, penWidth) End If Return rect End Function ''' <summary> ''' Draws the object on specified <see cref="System.Drawing.Graphics" /> in the object space. ''' </summary> ''' <param name="viewer">An image viewer.</param> ''' <param name="g">A graphics where the object must be drawn.</param> ''' <remarks> ''' This method draws object after the PointTransform is applied to ''' the Graphics, specified by <i>g</i> parameter.<br /><br /> ''' By default this method does not do anything. ''' </remarks> Public Overrides Sub DrawInObjectSpace(viewer As Vintasoft.Imaging.UI.ImageViewer, g As System.Drawing.Graphics) If viewer.Image Is Nothing Then Return End If Dim rect As System.Drawing.RectangleF = GetRectangle(_location, viewer.Image) If Brush IsNot Nothing Then g.FillRectangle(Brush, rect) End If If Pen IsNot Nothing AndAlso Not CellSize.IsEmpty Then Dim cellHeight As Single = CSng(Vintasoft.Imaging.Utils.UnitOfMeasureConverter.ConvertToPixels(CellSize.Height, CellUnitOfMeasure)) Dim countRow As Integer = CInt(Math.Truncate(System.Math.Ceiling(rect.Height / cellHeight))) Dim cellWidth As Single = CSng(Vintasoft.Imaging.Utils.UnitOfMeasureConverter.ConvertToPixels(CellSize.Width, CellUnitOfMeasure)) Dim countColumn As Integer = CInt(Math.Truncate(System.Math.Ceiling(rect.Width / cellWidth))) If countRow > 0 AndAlso countColumn > 0 Then Dim x1 As Single = rect.X Dim y1 As Single = rect.Y + cellHeight Dim x2 As Single = rect.Right Dim i As Integer i = 1 While i < countRow g.DrawLine(Pen, x1, y1, x2, y1) i += 1 y1 += cellHeight End While x1 += cellWidth y1 = rect.Y Dim y2 As Single = rect.Bottom i = 1 While i < countColumn g.DrawLine(Pen, x1, y1, x1, y2) i += 1 x1 += cellWidth End While End If End If End Sub ''' <summary> ''' Creates a new WpfGridGraphicObject that is a copy of the current instance. ''' </summary> ''' <returns> ''' A new WpfGridGraphicObject that is a copy of this instance. ''' </returns> Public Overrides Function Clone() As Object Dim obj As New GridGraphicObject(_tool) CopyTo(obj) Return obj End Function ''' <summary> ''' Copies current WpfGridGraphicObject to the target WpfGridGraphicObject. ''' </summary> ''' <param name="obj">WpfGridGraphicObject to copy.</param> Public Overrides Sub CopyTo(obj As Vintasoft.Imaging.UI.VisualTools.GraphicObjects.GraphicObject) MyBase.CopyTo(obj) Dim gridObj As GridGraphicObject = TryCast(obj, GridGraphicObject) If gridObj IsNot Nothing Then gridObj.Location = Location gridObj.CellSize = CellSize gridObj.CellUnitOfMeasure = CellUnitOfMeasure End If End Sub ''' <summary> ''' Gets the rectangle of grid. ''' </summary> ''' <param name="location">The location.</param> ''' <param name="image">The image.</param> Private Function GetRectangle(location As System.Drawing.PointF, image As Vintasoft.Imaging.VintasoftImage) As System.Drawing.RectangleF Dim rect As New System.Drawing.RectangleF(_location.X, _location.Y, image.Width - _location.X, image.Height - _location.Y) Return rect End Function #End Region End Class
/// <summary> /// Displays grid over image in image viewer. /// </summary> /// <param name="viewer">The viewer.</param> public static void DrawGridOverImageInImageViewer(Vintasoft.Imaging.UI.ImageViewer viewer) { // create visual tool that can display static graphic objects in an image viewer Vintasoft.Imaging.UI.VisualTools.GraphicObjects.GraphicObjectTool tool = new Vintasoft.Imaging.UI.VisualTools.GraphicObjects.GraphicObjectTool(); // create graphic object that displays image grid GridGraphicObject grid = new GridGraphicObject(tool); // add graphic object to the visual tool tool.GraphicObjectCollection.Add(grid); // set visual tool as current visual tool of image viewer viewer.VisualTool = tool; } /// <summary> /// Represents an image grid which can be displayed in image viewer. /// </summary> public class GridGraphicObject : Vintasoft.Imaging.UI.VisualTools.GraphicObjects.GraphicObject { #region Fields Vintasoft.Imaging.UI.VisualTools.VisualTool _tool; #endregion #region Constructors /// <summary> /// Initializes a new instance of the <see cref="GridGraphicObject"/> class. /// </summary> /// <param name="tool">The visual tool.</param> public GridGraphicObject(Vintasoft.Imaging.UI.VisualTools.VisualTool tool) { if (tool == null) throw new System.ArgumentNullException(); _tool = tool; Pen = new System.Drawing.Pen( System.Drawing.Color.FromArgb(128, System.Drawing.Color.Lime), 1); PointTransform = new Vintasoft.Imaging.UI.VisualTools.PixelsToImageViewerPointFTransform(); } #endregion #region Properties System.Drawing.PointF _location = System.Drawing.PointF.Empty; /// <summary> /// Gets or sets the location of grid. /// </summary> /// <value> /// Default value is <b>(0,0)</b>. /// </value> public System.Drawing.PointF Location { get { return _location; } set { _location = value; } } System.Drawing.SizeF _cellSize = new System.Drawing.SizeF(1, 1); /// <summary> /// Gets or sets the size of the grid cell. /// </summary> /// <value> /// Default value is <b>(1,1)</b>. /// </value> public System.Drawing.SizeF CellSize { get { return _cellSize; } set { _cellSize = value; } } Vintasoft.Imaging.UnitOfMeasure _cellUnitOfMeasure = Vintasoft.Imaging.UnitOfMeasure.Centimeters; /// <summary> /// Gets or sets the unit of measure of the grid cell. /// </summary> /// <value> /// Default value is <b>UnitOfMeasure.Centimeters</b>. /// </value> public Vintasoft.Imaging.UnitOfMeasure CellUnitOfMeasure { get { return _cellUnitOfMeasure; } set { _cellUnitOfMeasure = value; } } #endregion #region Methods /// <summary> /// Returns a value indicating whether point belongs the object. /// </summary> /// <param name="x">X coordinate of point in object space.</param> /// <param name="y">Y coordinate of point in object space.</param> /// <returns> /// <b>true</b> if point belongs the object; /// otherwise, <b>false</b>. /// </returns> public override bool IsPointOnObject(float x, float y) { return IsPointOnObject(x, y, false); } /// <summary> /// Returns a value indicating whether point belongs the object. /// </summary> /// <param name="x">X coordinate of point in object space.</param> /// <param name="y">Y coordinate of point in object space.</param> /// <param name="ignoreContainmentCheckDistance">A value indicating whether the point must be checked on the object only (ignore /// the "containment" region around object that is used for object selection).</param> /// <returns> /// <b>true</b> if point belongs the object; /// otherwise, <b>false</b>. /// </returns> public override bool IsPointOnObject(float x, float y, bool ignoreContainmentCheckDistance) { if (_tool.ImageViewer.Image != null && (Pen != null || Brush != null)) { System.Drawing.RectangleF rect = GetRectangle(_location, _tool.ImageViewer.Image); return rect.Contains(x, y); } else return false; } /// <summary> /// Returns a bounding box of object, in object space. /// </summary> /// <returns> /// Bounding box of object, in object space. /// </returns> public override System.Drawing.RectangleF GetBoundingBox() { if (_tool.ImageViewer.Image != null) return GetRectangle(_location, _tool.ImageViewer.Image); else return System.Drawing.RectangleF.Empty; } /// <summary> /// Returns a drawing rectangle of object, in object space. /// </summary> /// <param name="viewer">An image viewer.</param> /// <returns> /// Drawing rectangle of object, in object space. /// </returns> public override System.Drawing.RectangleF GetDrawingBox(Vintasoft.Imaging.UI.ImageViewer viewer) { if (viewer.Image == null) return System.Drawing.RectangleF.Empty; System.Drawing.RectangleF rect = GetRectangle(_location, viewer.Image); if (Pen != null) { float penWidth = Pen.Width / 2.0f; rect.Inflate(penWidth, penWidth); } return rect; } /// <summary> /// Draws the object on specified <see cref="System.Drawing.Graphics" /> in the object space. /// </summary> /// <param name="viewer">An image viewer.</param> /// <param name="g">A graphics where the object must be drawn.</param> /// <remarks> /// This method draws object after the PointTransform is applied to /// the Graphics, specified by <i>g</i> parameter.<br /><br /> /// By default this method does not do anything. /// </remarks> public override void DrawInObjectSpace(Vintasoft.Imaging.UI.ImageViewer viewer, System.Drawing.Graphics g) { if (viewer.Image == null) return; System.Drawing.RectangleF rect = GetRectangle(_location, viewer.Image); if (Brush != null) g.FillRectangle(Brush, rect); if (Pen != null && !CellSize.IsEmpty) { float cellHeight = (float)Vintasoft.Imaging.Utils.UnitOfMeasureConverter.ConvertToPixels(CellSize.Height, CellUnitOfMeasure); int countRow = (int)System.Math.Ceiling(rect.Height / cellHeight); float cellWidth = (float)Vintasoft.Imaging.Utils.UnitOfMeasureConverter.ConvertToPixels(CellSize.Width, CellUnitOfMeasure); int countColumn = (int)System.Math.Ceiling(rect.Width / cellWidth); if (countRow > 0 && countColumn > 0) { float x1 = rect.X; float y1 = rect.Y + cellHeight; float x2 = rect.Right; int i; for (i = 1; i < countRow; i++, y1 += cellHeight) g.DrawLine(Pen, x1, y1, x2, y1); x1 += cellWidth; y1 = rect.Y; float y2 = rect.Bottom; for (i = 1; i < countColumn; i++, x1 += cellWidth) g.DrawLine(Pen, x1, y1, x1, y2); } } } /// <summary> /// Creates a new WpfGridGraphicObject that is a copy of the current instance. /// </summary> /// <returns> /// A new WpfGridGraphicObject that is a copy of this instance. /// </returns> public override object Clone() { GridGraphicObject obj = new GridGraphicObject(_tool); CopyTo(obj); return obj; } /// <summary> /// Copies current WpfGridGraphicObject to the target WpfGridGraphicObject. /// </summary> /// <param name="obj">WpfGridGraphicObject to copy.</param> public override void CopyTo(Vintasoft.Imaging.UI.VisualTools.GraphicObjects.GraphicObject obj) { base.CopyTo(obj); GridGraphicObject gridObj = obj as GridGraphicObject; if (gridObj != null) { gridObj.Location = Location; gridObj.CellSize = CellSize; gridObj.CellUnitOfMeasure = CellUnitOfMeasure; } } /// <summary> /// Gets the rectangle of grid. /// </summary> /// <param name="location">The location.</param> /// <param name="image">The image.</param> private System.Drawing.RectangleF GetRectangle( System.Drawing.PointF location, Vintasoft.Imaging.VintasoftImage image) { System.Drawing.RectangleF rect = new System.Drawing.RectangleF( _location.X, _location.Y, image.Width - _location.X, image.Height - _location.Y); return rect; } #endregion }
System.Object
 Vintasoft.Imaging.UI.VisualTools.GraphicObjects.GraphicObject
   Vintasoft.Imaging.Dicom.UI.VisualTools.ImageRulerGraphicObject
   Vintasoft.Imaging.UI.VisualTools.GraphicObjects.RectangularGraphicObject
   Vintasoft.Imaging.UI.VisualTools.GraphicObjects.GraphicObjectGroup
   Vintasoft.Imaging.UI.VisualTools.GraphicObjects.PathGraphicObject
Target Platforms: .NET9; .NET 8; .NET 7; .NET 6; .NET Framework 4.8, 4.7, 4.6, 4.5, 4.0, 3.5