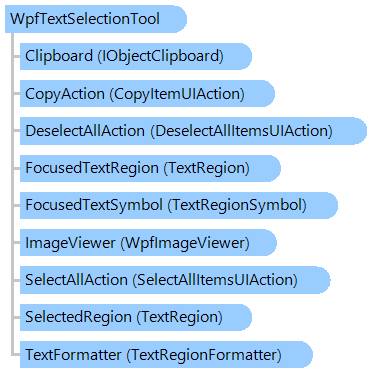
'Declaration <ToolboxItemAttribute("ToolboxItemType = null", "ToolboxItemTypeName = ")> <DesignTimeVisibleAttribute("Visible = False")> <DefaultPropertyAttribute("Content")> <ContentPropertyAttribute("Content")> <LocalizabilityAttribute(None)> <StyleTypedPropertyAttribute("Property = FocusVisualStyle", "StyleTargetType = System.Windows.Controls.Control")> <XmlLangPropertyAttribute("Name = Language")> <UsableDuringInitializationAttribute("Usable = True")> <RuntimeNamePropertyAttribute("Name = Name")> <UidPropertyAttribute()> <TypeDescriptionProviderAttribute("TypeName = MS.Internal.ComponentModel.DependencyObjectProvider")> <NameScopePropertyAttribute("Name = NameScope", "Type = System.Windows.NameScope")> Public Class WpfTextSelectionTool Inherits WpfVisualTool Implements ISupportUIActions
[ToolboxItem("ToolboxItemType = null", "ToolboxItemTypeName = ")] [DesignTimeVisible("Visible = False")] [DefaultProperty("Content")] [ContentProperty("Content")] [Localizability(None)] [StyleTypedProperty("Property = FocusVisualStyle", "StyleTargetType = System.Windows.Controls.Control")] [XmlLangProperty("Name = Language")] [UsableDuringInitialization("Usable = True")] [RuntimeNameProperty("Name = Name")] [UidProperty()] [TypeDescriptionProvider("TypeName = MS.Internal.ComponentModel.DependencyObjectProvider")] [NameScopeProperty("Name = NameScope", "Type = System.Windows.NameScope")] public class WpfTextSelectionTool : WpfVisualTool, ISupportUIActions
[ToolboxItem("ToolboxItemType = null", "ToolboxItemTypeName = ")] [DesignTimeVisible("Visible = False")] [DefaultProperty("Content")] [ContentProperty("Content")] [Localizability(None)] [StyleTypedProperty("Property = FocusVisualStyle", "StyleTargetType = System.Windows.Controls.Control")] [XmlLangProperty("Name = Language")] [UsableDuringInitialization("Usable = True")] [RuntimeNameProperty("Name = Name")] [UidProperty()] [TypeDescriptionProvider("TypeName = MS.Internal.ComponentModel.DependencyObjectProvider")] [NameScopeProperty("Name = NameScope", "Type = System.Windows.NameScope")] public __gc class WpfTextSelectionTool : public WpfVisualTool*, ISupportUIActions
[ToolboxItem("ToolboxItemType = null", "ToolboxItemTypeName = ")] [DesignTimeVisible("Visible = False")] [DefaultProperty("Content")] [ContentProperty("Content")] [Localizability(None)] [StyleTypedProperty("Property = FocusVisualStyle", "StyleTargetType = System.Windows.Controls.Control")] [XmlLangProperty("Name = Language")] [UsableDuringInitialization("Usable = True")] [RuntimeNameProperty("Name = Name")] [UidProperty()] [TypeDescriptionProvider("TypeName = MS.Internal.ComponentModel.DependencyObjectProvider")] [NameScopeProperty("Name = NameScope", "Type = System.Windows.NameScope")] public ref class WpfTextSelectionTool : public WpfVisualTool^, ISupportUIActions
This C#/VB.NET code shows how to search text in document loaded into WPF image viewer.
System.Object
 System.Windows.Threading.DispatcherObject
   System.Windows.DependencyObject
     System.Windows.Media.Visual
       System.Windows.UIElement
         System.Windows.FrameworkElement
           System.Windows.Controls.Control
             System.Windows.Controls.ContentControl
               Vintasoft.Imaging.Wpf.UI.VisualTools.WpfVisualTool
                 Vintasoft.Imaging.Wpf.UI.VisualTools.WpfTextSelectionTool
                   Vintasoft.Imaging.Office.OpenXml.Wpf.UI.VisualTools.UserInteraction.WpfOfficeDocumentVisualEditorTextTool
Target Platforms: .NET9; .NET 8; .NET 7; .NET 6; .NET Framework 4.8, 4.7, 4.6, 4.5, 4.0, 3.5
WpfTextSelectionTool Members
Vintasoft.Imaging.Wpf.UI.VisualTools Namespace
IsKeyboardSelectionEnabled
IsMouseSelectionEnabled