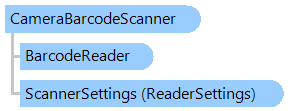
'Declaration Public Class CameraBarcodeScanner
public class CameraBarcodeScanner
public __gc class CameraBarcodeScanner
public ref class CameraBarcodeScanner
Class provides the optimal CPU load and the best barcode recognition speed when barcodes are recognized in images from a streaming video.
This C#/VB.NET code shows how to capture images from DirectShow camera (VintaSoft Imaging .NET SDK) and recognize barcodes in captured images (VintaSoft Barcode .NET SDK).
Public Partial Class BarcodeScannerExampleForm Inherits Form ''' <summary> ''' The camera barcode scanner. ''' </summary> Private _cameraBarcodeScanner As ImagingCameraBarcodeScanner ''' <summary> ''' Initializes a new instance of the <see cref="BarcodeScannerExampleForm"/> class. ''' </summary> Public Sub New() InitializeComponent() _cameraBarcodeScanner = New ImagingCameraBarcodeScanner() AddHandler _cameraBarcodeScanner.BarcodeScanner.FrameScanFinished, AddressOf BarcodeScanner_FrameScanFinished AddHandler _cameraBarcodeScanner.ScanningException, AddressOf CameraBarcodeScanner_ScanningException _cameraBarcodeScanner.BarcodeScanner.ScannerSettings.ScanBarcodeTypes = Vintasoft.Barcode.BarcodeType.QR End Sub ''' <summary> ''' Starts the capturing of images from seleced device and recognition of barcodes in captured images. ''' </summary> Private Sub startButton_Click(sender As Object, e As EventArgs) textBox1.Text = "Scan Barcodes Types: " & _cameraBarcodeScanner.BarcodeScanner.ScannerSettings.ScanBarcodeTypes.ToString() _cameraBarcodeScanner.CaptureDevice = _cameraBarcodeScanner.CaptureDevices(0) _cameraBarcodeScanner.StartScanning() End Sub ''' <summary> ''' Stops the capturing of images from seleced device and recognition of barcodes in captured images. ''' </summary> Private Sub stopButton_Click(sender As Object, e As EventArgs) textBox1.Text = "" _cameraBarcodeScanner.StopScanning() End Sub ''' <summary> ''' Shows an error. ''' </summary> Private Sub CameraBarcodeScanner_ScanningException(sender As Object, e As Vintasoft.Imaging.ExceptionEventArgs) MessageBox.Show(e.Exception.ToString()) End Sub ''' <summary> ''' Handles the FrameScanFinished event of the BarcodeScanner. ''' </summary> Private Sub BarcodeScanner_FrameScanFinished(sender As Object, e As Vintasoft.Barcode.FrameScanFinishedEventArgs) If e.FoundBarcodes.Length > 0 Then Invoke(New ShowRecognitionResultsDelegate(AddressOf ShowRecognitionResults), New Object() {e.FoundBarcodes}) End If End Sub ''' <summary> ''' Shows barcode recognition results. ''' </summary> Private Sub ShowRecognitionResults(barcodes As Vintasoft.Barcode.IBarcodeInfo()) Dim result As New StringBuilder() For Each barcode As Vintasoft.Barcode.IBarcodeInfo In barcodes result.AppendLine(String.Format("{0}: {1}", barcode.BarcodeType, barcode.Value)) Next ' show barcode recognition in text box textBox1.Text = result.ToString() End Sub Private Delegate Sub ShowRecognitionResultsDelegate(barcodeInfo As Vintasoft.Barcode.IBarcodeInfo()) End Class ''' <summary> ''' Allows to capture images from DirectShow camera (VintaSoft Imaging .NET SDK) and recognize barcodes in captured images (VintaSoft Barcode .NET SDK). ''' </summary> Public Class ImagingCameraBarcodeScanner Implements IDisposable #Region "Fields" ''' <summary> ''' Monitor for capture devices. ''' </summary> Private _captureDevicesMonitor As Vintasoft.Imaging.Media.ImageCaptureDevicesMonitor ''' <summary> ''' Image capture source for barcode recognition. ''' </summary> Private _imageCaptureSource As Vintasoft.Imaging.Media.ImageCaptureSource #End Region #Region "Constructors" ''' <summary> ''' Initializes a new instance of the <see cref="ImagingCameraBarcodeScanner"/> class. ''' </summary> Public Sub New() _barcodeScanner = New Vintasoft.Barcode.CameraBarcodeScanner() AddHandler _barcodeScanner.FrameScanException, AddressOf BarcodeScanner_FrameScanException _barcodeScanner.ScannerSettings.ScanBarcodeTypes = Vintasoft.Barcode.BarcodeType.Code39 Or Vintasoft.Barcode.BarcodeType.Code128 Or Vintasoft.Barcode.BarcodeType.QR Or Vintasoft.Barcode.BarcodeType.DataMatrix _captureDevicesMonitor = New Vintasoft.Imaging.Media.ImageCaptureDevicesMonitor() AddHandler _captureDevicesMonitor.CaptureDevicesChanged, AddressOf CaptureDevicesMonitor_CaptureDevicesChanged If Not Vintasoft.Imaging.ImagingEnvironment.IsInDesignMode Then _captureDevicesMonitor.Start() End If _imageCaptureSource = New Vintasoft.Imaging.Media.ImageCaptureSource() AddHandler _imageCaptureSource.CaptureCompleted, AddressOf ImageCaptureSource_CaptureCompleted End Sub #End Region #Region "Properties" Private _captureDevice As Vintasoft.Imaging.Media.ImageCaptureDevice ''' <summary> ''' Gets or sets current capture device. ''' </summary> Public Property CaptureDevice() As Vintasoft.Imaging.Media.ImageCaptureDevice Get Return _captureDevice End Get Set If _captureDevice IsNot value Then _captureDevice = value UpdateCapureFormats() End If End Set End Property Private _captureFormats As ReadOnlyCollection(Of Vintasoft.Imaging.Media.ImageCaptureFormat) = Nothing ''' <summary> ''' Gets the available capture formats of current capture device. ''' </summary> ''' <seealso cref="CaptureDevice"/> Public ReadOnly Property CaptureFormats() As ReadOnlyCollection(Of Vintasoft.Imaging.Media.ImageCaptureFormat) Get Return _captureFormats End Get End Property ''' <summary> ''' Gets available capture devices. ''' </summary> Public ReadOnly Property CaptureDevices() As ReadOnlyCollection(Of Vintasoft.Imaging.Media.ImageCaptureDevice) Get Return Vintasoft.Imaging.Media.ImageCaptureDeviceConfiguration.GetCaptureDevices() End Get End Property Private _barcodeScanner As Vintasoft.Barcode.CameraBarcodeScanner ''' <summary> ''' Gets the barcode scanner. ''' </summary> Public ReadOnly Property BarcodeScanner() As Vintasoft.Barcode.CameraBarcodeScanner Get Return _barcodeScanner End Get End Property ''' <summary> ''' Gets a value indicating whether barcode scanning is started. ''' </summary> Public ReadOnly Property IsStarted() As Boolean Get Return _imageCaptureSource.State = Vintasoft.Imaging.Media.ImageCaptureState.Started End Get End Property #End Region #Region "Methods" #Region "PUBLIC" ''' <summary> ''' Starts the capturing of images from seleced device and recognition of barcodes in captured images. ''' </summary> Public Sub StartScanning() Try If CaptureDevice Is Nothing Then Throw New ArgumentNullException("CaptureDevice") End If If CaptureFormats Is Nothing OrElse CaptureFormats.Count = 0 Then Throw New ArgumentNullException("CaptureFormats") End If If _imageCaptureSource.State <> Vintasoft.Imaging.Media.ImageCaptureState.Started Then If CaptureDevice.DesiredFormat Is Nothing Then CaptureDevice.DesiredFormat = CaptureFormats(0) End If _barcodeScanner.StartScanning() _imageCaptureSource.CaptureDevice = _captureDevice _imageCaptureSource.Start() _imageCaptureSource.CaptureAsync() OnScanningStart(EventArgs.Empty) End If Catch ex As Exception OnScanningException(New Vintasoft.Imaging.ExceptionEventArgs(ex)) End Try End Sub ''' <summary> ''' Stops the capturing of images from seleced device and recognition of barcodes in captured images. ''' </summary> Public Sub StopScanning() Try If _imageCaptureSource.State <> Vintasoft.Imaging.Media.ImageCaptureState.Stopped Then _barcodeScanner.StopScanning() _imageCaptureSource.[Stop]() OnScanningStop(EventArgs.Empty) End If Catch ex As Exception OnScanningException(New Vintasoft.Imaging.ExceptionEventArgs(ex)) End Try End Sub ''' <summary> ''' Performs application-defined tasks associated with freeing, releasing, or resetting unmanaged resources. ''' </summary> Public Sub Dispose() Implements IDisposable.Dispose RemoveHandler _captureDevicesMonitor.CaptureDevicesChanged, AddressOf CaptureDevicesMonitor_CaptureDevicesChanged _barcodeScanner.BarcodeReader.Dispose() End Sub #End Region #Region "PROTECTED" ''' <summary> ''' Raises the <see cref="CaptureDevicesChanged" /> event. ''' </summary> ''' <param name="args">The <see cref="ImageCaptureDevicesChangedEventArgs"/> instance containing the event data.</param> Protected Overridable Sub OnCaptureDevicesChanged(args As Vintasoft.Imaging.Media.ImageCaptureDevicesChangedEventArgs) RaiseEvent CaptureDevicesChanged(Me, args) End Sub ''' <summary> ''' Raises the <see cref="ScanningException" /> event. ''' </summary> ''' <param name="args">The <see cref="ExceptionEventArgs"/> instance containing the event data.</param> Protected Overridable Sub OnScanningException(args As Vintasoft.Imaging.ExceptionEventArgs) RaiseEvent ScanningException(Me, args) End Sub ''' <summary> ''' Raises the <see cref="ScanningStart" /> event. ''' </summary> ''' <param name="args">The <see cref="EventArgs"/> instance containing the event data.</param> Protected Overridable Sub OnScanningStart(args As EventArgs) RaiseEvent ScanningStart(Me, args) End Sub ''' <summary> ''' Raises the <see cref="ScanningStop" /> event. ''' </summary> ''' <param name="args">The <see cref="EventArgs"/> instance containing the event data.</param> Protected Overridable Sub OnScanningStop(args As EventArgs) RaiseEvent ScanningStop(Me, args) End Sub #End Region #Region "PRIVATE" ''' <summary> ''' Handles the FrameScanException event of the BarcodeScanner control. ''' </summary> ''' <param name="sender">The source of the event.</param> ''' <param name="e">The <see cref="Vintasoft.Barcode.FrameScanExceptionEventArgs"/> instance containing the event data.</param> Private Sub BarcodeScanner_FrameScanException(sender As Object, e As Vintasoft.Barcode.FrameScanExceptionEventArgs) OnScanningException(New Vintasoft.Imaging.ExceptionEventArgs(e.Exception)) End Sub ''' <summary> ''' Handles the CaptureCompleted event of the ImageCaptureSource. ''' </summary> ''' <param name="sender">The source of the event.</param> ''' <param name="e">The <see cref="ImageCaptureCompletedEventArgs"/> instance containing the event data.</param> Private Sub ImageCaptureSource_CaptureCompleted(sender As Object, e As Vintasoft.Imaging.Media.ImageCaptureCompletedEventArgs) Try ' get captured image Dim image As Vintasoft.Imaging.VintasoftImage = e.GetCapturedImage() ' recognize barcodes from captured image Using bitmap As Vintasoft.Imaging.VintasoftBitmap = image.GetAsVintasoftBitmap() _barcodeScanner.ScanFrame(bitmap) End Using ' if image capturing is started If _imageCaptureSource.State = Vintasoft.Imaging.Media.ImageCaptureState.Started Then ' capture next image _imageCaptureSource.CaptureAsync() End If Catch ex As Exception OnScanningException(New Vintasoft.Imaging.ExceptionEventArgs(ex)) End Try End Sub ''' <summary> ''' Handles the CaptureDevicesChanged event of the CaptureDevicesMonitor. ''' </summary> ''' <param name="sender">The source of the event.</param> ''' <param name="e">The <see cref="ImageCaptureDevicesChangedEventArgs"/> instance containing the event data.</param> Private Sub CaptureDevicesMonitor_CaptureDevicesChanged(sender As Object, e As Vintasoft.Imaging.Media.ImageCaptureDevicesChangedEventArgs) If Array.IndexOf(e.RemovedDevices, CaptureDevice) >= 0 Then StopScanning() End If OnCaptureDevicesChanged(e) End Sub ''' <summary> ''' Updates the capture formats. ''' </summary> Private Sub UpdateCapureFormats() Dim device As Vintasoft.Imaging.Media.ImageCaptureDevice = CaptureDevice If device Is Nothing OrElse device.SupportedFormats Is Nothing Then _captureFormats = Nothing Else Dim captureFormats As New List(Of Vintasoft.Imaging.Media.ImageCaptureFormat)() Dim imageCaptureFormatSizes As New List(Of UInteger)() For i As Integer = 0 To device.SupportedFormats.Count - 1 ' if format has bit depth less or equal than 12 bit If device.SupportedFormats(i).BitsPerPixel <= 12 Then ' ignore formats with bit depth less or equal than 12 bit because they may cause issues on Windows 8 Continue For End If Dim imageCaptureFormatSize As UInteger = CUInt(device.SupportedFormats(i).Width Or (device.SupportedFormats(i).Height << 16)) If Not imageCaptureFormatSizes.Contains(imageCaptureFormatSize) Then imageCaptureFormatSizes.Add(imageCaptureFormatSize) captureFormats.Add(device.SupportedFormats(i)) End If Next _captureFormats = captureFormats.AsReadOnly() End If End Sub #End Region #End Region #Region "Events" ''' <summary> ''' Occurs when barcode scanning is started. ''' </summary> Public Event ScanningStart As EventHandler ''' <summary> ''' Occurs when barcode scanning is stopped. ''' </summary> Public Event ScanningStop As EventHandler ''' <summary> ''' Occurs when barcode scanning error occurs. ''' </summary> Public Event ScanningException As EventHandler(Of Vintasoft.Imaging.ExceptionEventArgs) ''' <summary> ''' Occurs when <see cref="CaptureDevices"/> property is changed. ''' </summary> Public Event CaptureDevicesChanged As EventHandler(Of Vintasoft.Imaging.Media.ImageCaptureDevicesChangedEventArgs) #End Region End Class
public partial class BarcodeScannerExampleForm : Form { /// <summary> /// The camera barcode scanner. /// </summary> ImagingCameraBarcodeScanner _cameraBarcodeScanner; /// <summary> /// Initializes a new instance of the <see cref="BarcodeScannerExampleForm"/> class. /// </summary> public BarcodeScannerExampleForm() { InitializeComponent(); _cameraBarcodeScanner = new ImagingCameraBarcodeScanner(); _cameraBarcodeScanner.BarcodeScanner.FrameScanFinished += BarcodeScanner_FrameScanFinished; _cameraBarcodeScanner.ScanningException += CameraBarcodeScanner_ScanningException; _cameraBarcodeScanner.BarcodeScanner.ScannerSettings.ScanBarcodeTypes = Vintasoft.Barcode.BarcodeType.QR; } /// <summary> /// Starts the capturing of images from seleced device and recognition of barcodes in captured images. /// </summary> private void startButton_Click(object sender, EventArgs e) { textBox1.Text = "Scan Barcodes Types: " + _cameraBarcodeScanner.BarcodeScanner.ScannerSettings.ScanBarcodeTypes.ToString(); _cameraBarcodeScanner.CaptureDevice = _cameraBarcodeScanner.CaptureDevices[0]; _cameraBarcodeScanner.StartScanning(); } /// <summary> /// Stops the capturing of images from seleced device and recognition of barcodes in captured images. /// </summary> private void stopButton_Click(object sender, EventArgs e) { textBox1.Text = ""; _cameraBarcodeScanner.StopScanning(); } /// <summary> /// Shows an error. /// </summary> private void CameraBarcodeScanner_ScanningException(object sender, Vintasoft.Imaging.ExceptionEventArgs e) { MessageBox.Show(e.Exception.ToString()); } /// <summary> /// Handles the FrameScanFinished event of the BarcodeScanner. /// </summary> private void BarcodeScanner_FrameScanFinished(object sender, Vintasoft.Barcode.FrameScanFinishedEventArgs e) { if (e.FoundBarcodes.Length > 0) Invoke(new ShowRecognitionResultsDelegate(ShowRecognitionResults), new object[] { e.FoundBarcodes }); } /// <summary> /// Shows barcode recognition results. /// </summary> private void ShowRecognitionResults(Vintasoft.Barcode.IBarcodeInfo[] barcodes) { StringBuilder result = new StringBuilder(); foreach (Vintasoft.Barcode.IBarcodeInfo barcode in barcodes) result.AppendLine(string.Format("{0}: {1}", barcode.BarcodeType, barcode.Value)); // show barcode recognition in text box textBox1.Text = result.ToString(); } delegate void ShowRecognitionResultsDelegate(Vintasoft.Barcode.IBarcodeInfo[] barcodeInfo); } /// <summary> /// Allows to capture images from DirectShow camera (VintaSoft Imaging .NET SDK) and recognize barcodes in captured images (VintaSoft Barcode .NET SDK). /// </summary> public class ImagingCameraBarcodeScanner : IDisposable { #region Fields /// <summary> /// Monitor for capture devices. /// </summary> Vintasoft.Imaging.Media.ImageCaptureDevicesMonitor _captureDevicesMonitor; /// <summary> /// Image capture source for barcode recognition. /// </summary> Vintasoft.Imaging.Media.ImageCaptureSource _imageCaptureSource; #endregion #region Constructors /// <summary> /// Initializes a new instance of the <see cref="ImagingCameraBarcodeScanner"/> class. /// </summary> public ImagingCameraBarcodeScanner() { _barcodeScanner = new Vintasoft.Barcode.CameraBarcodeScanner(); _barcodeScanner.FrameScanException += BarcodeScanner_FrameScanException; _barcodeScanner.ScannerSettings.ScanBarcodeTypes = Vintasoft.Barcode.BarcodeType.Code39 | Vintasoft.Barcode.BarcodeType.Code128 | Vintasoft.Barcode.BarcodeType.QR | Vintasoft.Barcode.BarcodeType.DataMatrix; _captureDevicesMonitor = new Vintasoft.Imaging.Media.ImageCaptureDevicesMonitor(); _captureDevicesMonitor.CaptureDevicesChanged += CaptureDevicesMonitor_CaptureDevicesChanged; if (!Vintasoft.Imaging.ImagingEnvironment.IsInDesignMode) _captureDevicesMonitor.Start(); _imageCaptureSource = new Vintasoft.Imaging.Media.ImageCaptureSource(); _imageCaptureSource.CaptureCompleted += ImageCaptureSource_CaptureCompleted; } #endregion #region Properties Vintasoft.Imaging.Media.ImageCaptureDevice _captureDevice; /// <summary> /// Gets or sets current capture device. /// </summary> public Vintasoft.Imaging.Media.ImageCaptureDevice CaptureDevice { get { return _captureDevice; } set { if (_captureDevice != value) { _captureDevice = value; UpdateCapureFormats(); } } } ReadOnlyCollection<Vintasoft.Imaging.Media.ImageCaptureFormat> _captureFormats = null; /// <summary> /// Gets the available capture formats of current capture device. /// </summary> /// <seealso cref="CaptureDevice"/> public ReadOnlyCollection<Vintasoft.Imaging.Media.ImageCaptureFormat> CaptureFormats { get { return _captureFormats; } } /// <summary> /// Gets available capture devices. /// </summary> public ReadOnlyCollection<Vintasoft.Imaging.Media.ImageCaptureDevice> CaptureDevices { get { return Vintasoft.Imaging.Media.ImageCaptureDeviceConfiguration.GetCaptureDevices(); } } Vintasoft.Barcode.CameraBarcodeScanner _barcodeScanner; /// <summary> /// Gets the barcode scanner. /// </summary> public Vintasoft.Barcode.CameraBarcodeScanner BarcodeScanner { get { return _barcodeScanner; } } /// <summary> /// Gets a value indicating whether barcode scanning is started. /// </summary> public bool IsStarted { get { return _imageCaptureSource.State == Vintasoft.Imaging.Media.ImageCaptureState.Started; } } #endregion #region Methods #region PUBLIC /// <summary> /// Starts the capturing of images from seleced device and recognition of barcodes in captured images. /// </summary> public void StartScanning() { try { if (CaptureDevice == null) throw new ArgumentNullException("CaptureDevice"); if (CaptureFormats == null || CaptureFormats.Count == 0) throw new ArgumentNullException("CaptureFormats"); if (_imageCaptureSource.State != Vintasoft.Imaging.Media.ImageCaptureState.Started) { if (CaptureDevice.DesiredFormat == null) CaptureDevice.DesiredFormat = CaptureFormats[0]; _barcodeScanner.StartScanning(); _imageCaptureSource.CaptureDevice = _captureDevice; _imageCaptureSource.Start(); _imageCaptureSource.CaptureAsync(); OnScanningStart(EventArgs.Empty); } } catch (Exception ex) { OnScanningException(new Vintasoft.Imaging.ExceptionEventArgs(ex)); } } /// <summary> /// Stops the capturing of images from seleced device and recognition of barcodes in captured images. /// </summary> public void StopScanning() { try { if (_imageCaptureSource.State != Vintasoft.Imaging.Media.ImageCaptureState.Stopped) { _barcodeScanner.StopScanning(); _imageCaptureSource.Stop(); OnScanningStop(EventArgs.Empty); } } catch (Exception ex) { OnScanningException(new Vintasoft.Imaging.ExceptionEventArgs(ex)); } } /// <summary> /// Performs application-defined tasks associated with freeing, releasing, or resetting unmanaged resources. /// </summary> public void Dispose() { _captureDevicesMonitor.CaptureDevicesChanged -= CaptureDevicesMonitor_CaptureDevicesChanged; _barcodeScanner.BarcodeReader.Dispose(); } #endregion #region PROTECTED /// <summary> /// Raises the <see cref="CaptureDevicesChanged" /> event. /// </summary> /// <param name="args">The <see cref="ImageCaptureDevicesChangedEventArgs"/> instance containing the event data.</param> protected virtual void OnCaptureDevicesChanged(Vintasoft.Imaging.Media.ImageCaptureDevicesChangedEventArgs args) { if (CaptureDevicesChanged != null) CaptureDevicesChanged(this, args); } /// <summary> /// Raises the <see cref="ScanningException" /> event. /// </summary> /// <param name="args">The <see cref="ExceptionEventArgs"/> instance containing the event data.</param> protected virtual void OnScanningException(Vintasoft.Imaging.ExceptionEventArgs args) { if (ScanningException != null) ScanningException(this, args); } /// <summary> /// Raises the <see cref="ScanningStart" /> event. /// </summary> /// <param name="args">The <see cref="EventArgs"/> instance containing the event data.</param> protected virtual void OnScanningStart(EventArgs args) { if (ScanningStart != null) ScanningStart(this, args); } /// <summary> /// Raises the <see cref="ScanningStop" /> event. /// </summary> /// <param name="args">The <see cref="EventArgs"/> instance containing the event data.</param> protected virtual void OnScanningStop(EventArgs args) { if (ScanningStop != null) ScanningStop(this, args); } #endregion #region PRIVATE /// <summary> /// Handles the FrameScanException event of the BarcodeScanner control. /// </summary> /// <param name="sender">The source of the event.</param> /// <param name="e">The <see cref="Vintasoft.Barcode.FrameScanExceptionEventArgs"/> instance containing the event data.</param> private void BarcodeScanner_FrameScanException(object sender, Vintasoft.Barcode.FrameScanExceptionEventArgs e) { OnScanningException(new Vintasoft.Imaging.ExceptionEventArgs(e.Exception)); } /// <summary> /// Handles the CaptureCompleted event of the ImageCaptureSource. /// </summary> /// <param name="sender">The source of the event.</param> /// <param name="e">The <see cref="ImageCaptureCompletedEventArgs"/> instance containing the event data.</param> private void ImageCaptureSource_CaptureCompleted(object sender, Vintasoft.Imaging.Media.ImageCaptureCompletedEventArgs e) { try { // get captured image Vintasoft.Imaging.VintasoftImage image = e.GetCapturedImage(); // recognize barcodes from captured image using (Vintasoft.Imaging.VintasoftBitmap bitmap = image.GetAsVintasoftBitmap()) _barcodeScanner.ScanFrame(bitmap); // if image capturing is started if (_imageCaptureSource.State == Vintasoft.Imaging.Media.ImageCaptureState.Started) { // capture next image _imageCaptureSource.CaptureAsync(); } } catch (Exception ex) { OnScanningException(new Vintasoft.Imaging.ExceptionEventArgs(ex)); } } /// <summary> /// Handles the CaptureDevicesChanged event of the CaptureDevicesMonitor. /// </summary> /// <param name="sender">The source of the event.</param> /// <param name="e">The <see cref="ImageCaptureDevicesChangedEventArgs"/> instance containing the event data.</param> private void CaptureDevicesMonitor_CaptureDevicesChanged(object sender, Vintasoft.Imaging.Media.ImageCaptureDevicesChangedEventArgs e) { if (Array.IndexOf(e.RemovedDevices, CaptureDevice) >= 0) { StopScanning(); } OnCaptureDevicesChanged(e); } /// <summary> /// Updates the capture formats. /// </summary> private void UpdateCapureFormats() { Vintasoft.Imaging.Media.ImageCaptureDevice device = CaptureDevice; if (device == null || device.SupportedFormats == null) { _captureFormats = null; } else { List<Vintasoft.Imaging.Media.ImageCaptureFormat> captureFormats = new List<Vintasoft.Imaging.Media.ImageCaptureFormat>(); List<uint> imageCaptureFormatSizes = new List<uint>(); for (int i = 0; i < device.SupportedFormats.Count; i++) { // if format has bit depth less or equal than 12 bit if (device.SupportedFormats[i].BitsPerPixel <= 12) { // ignore formats with bit depth less or equal than 12 bit because they may cause issues on Windows 8 continue; } uint imageCaptureFormatSize = (uint)(device.SupportedFormats[i].Width | (device.SupportedFormats[i].Height << 16)); if (!imageCaptureFormatSizes.Contains(imageCaptureFormatSize)) { imageCaptureFormatSizes.Add(imageCaptureFormatSize); captureFormats.Add(device.SupportedFormats[i]); } } _captureFormats = captureFormats.AsReadOnly(); } } #endregion #endregion #region Events /// <summary> /// Occurs when barcode scanning is started. /// </summary> public event EventHandler ScanningStart; /// <summary> /// Occurs when barcode scanning is stopped. /// </summary> public event EventHandler ScanningStop; /// <summary> /// Occurs when barcode scanning error occurs. /// </summary> public event EventHandler<Vintasoft.Imaging.ExceptionEventArgs> ScanningException; /// <summary> /// Occurs when <see cref="CaptureDevices"/> property is changed. /// </summary> public event EventHandler<Vintasoft.Imaging.Media.ImageCaptureDevicesChangedEventArgs> CaptureDevicesChanged; #endregion }
System.Object
 Vintasoft.Barcode.CameraBarcodeScanner
Target Platforms: .NET9; .NET 8; .NET 7; .NET 6; .NET Framework 4.8, 4.7, 4.6, 4.5, 4.0, 3.5