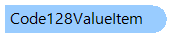
'Declaration Public Class Code128ValueItem Inherits TextValueItem
public class Code128ValueItem : TextValueItem
public __gc class Code128ValueItem : public TextValueItem*
public ref class Code128ValueItem : public TextValueItem^
System.Object
 Vintasoft.Barcode.BarcodeInfo.ValueItemBase
   Vintasoft.Barcode.BarcodeInfo.TextValueItem
     Vintasoft.Barcode.BarcodeInfo.Code128ValueItem
Target Platforms: .NET9; .NET 8; .NET 7; .NET 6; .NET Framework 4.8, 4.7, 4.6, 4.5, 4.0, 3.5