Add web image viewer to an ASP.NET Core application with React.js
In This Topic
This tutorial shows how to create a blank ASP.NET Core Web application in Visual Studio .NET 2022 and
add image viewer to ASP.NET Core application with React.js.
Here are steps, which must be done:
-
Create a blank ASP.NET Core Web application.
- Open Visual Studio .NET 2022 and create a new project, of ASP.NET Core Web application type:
- Configure the project to use .NET 6.0:
-
Server side: Add references to the Vintasoft assemblies to your ASP.NET Core Web application.
Copy Vintasoft.Shared.dll, Vintasoft.Imaging.dll, Vintasoft.Imaging.Pdf.dll,
Vintasoft.Imaging.Office.OpenXml, Vintasoft.Shared.Web.dll,
Vintasoft.Imaging.Web.Services.dll and Vintasoft.Imaging.AspNetCore.ApiControllers.dll assemblies
from "<SdkInstallPath>\VintaSoft Imaging .NET v12.4\Bin\DotNet6\AnyCPU\" directory to the "Bin" directory of
ASP.NET Core Web application and add references to assemblies in ASP.NET Core Web application.
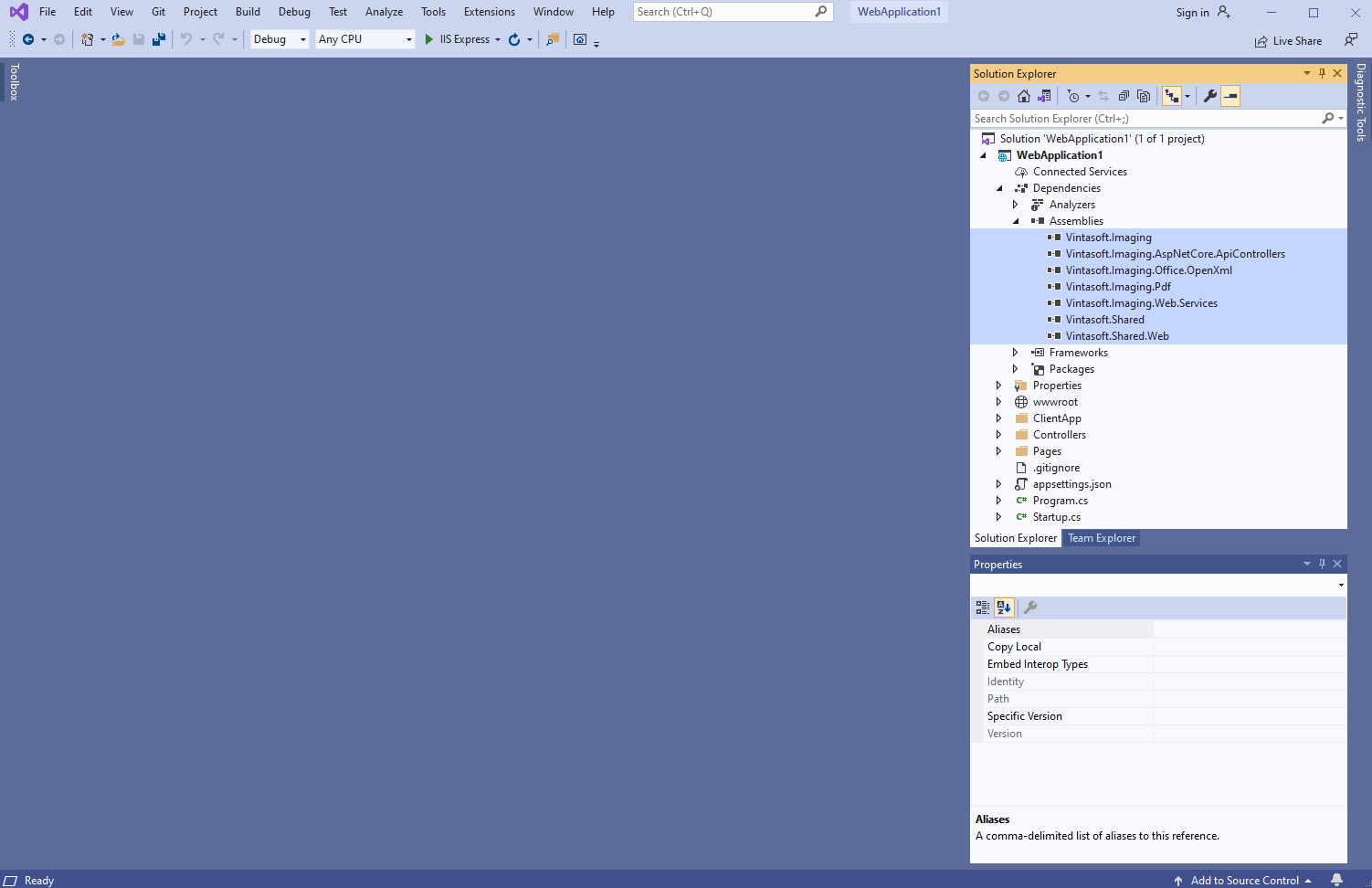
-
Server side: Specify drawing engine, which should be used by VintaSoft Imaging .NET SDK for drawing of 2D graphics.
If ASP.NET application must be used in Windows or Linux, SkiaSharp drawing engine should be used.
If ASP.NET application must be used in Windows only, System.Drawing or SkiaSharp drawing engine should be used.
Here are steps, which should be made for using SkiaSharp engine:
- Add reference to the Vintasoft.Imaging.Drawing.SkiaSharp.dll assembly.
- Add reference to the SkiaSharp nuget-package version 2.88.6.
- Open "Startup.cs" file, add code line "Vintasoft.Imaging.Drawing.SkiaSharp.SkiaSharpDrawingFactory.SetAsDefault();"
at the beginning of ConfigureServices method - added code specifies that VintaSoft Imaging .NET SDK should use
SkiaSharp library for drawing of 2D graphics.
Here are steps, which should be made for using System.Drawing engine:
- Add reference to the Vintasoft.Imaging.Gdi.dll assembly.
- Open "Startup.cs" file, add code line "Vintasoft.Imaging.Drawing.Gdi.GdiGraphicsFactory.SetAsDefault();"
at the beginning of ConfigureServices method - added code specifies that VintaSoft Imaging .NET SDK should use
System.Drawing library for drawing of 2D graphics.
-
Server side: Create web services, which allow to upload/download file, manage image collection, get information about images, get thumbnails and render image tiles.
-
Create web service that allows to upload/download file
- Press the right mouse button on the "Controllers" folder and select the "Add => Controller..." menu from context menu
- Select Empty API controller template, set the controller name to the "MyVintasoftFileApiController" and press the "Add" button
- Specify that MyVintasoftFileApiController class is derived from Vintasoft.Imaging.AspNetCore.ApiControllers.VintasoftFileApiController class
Here are source codes of MyVintasoftFileApiController class:
using Microsoft.AspNetCore.Hosting;
namespace WebApplication1.Controllers
{
public class MyVintasoftFileApiController : Vintasoft.Imaging.AspNetCore.ApiControllers.VintasoftFileApiController
{
public MyVintasoftFileApiController(IWebHostEnvironment hostingEnvironment)
: base(hostingEnvironment)
{
}
}
}
-
Create web service that allows to manage image collection
- Press the right mouse button on the "Controllers" folder and select the "Add => Controller..." menu from context menu
- Select Empty API controller template, set the controller name to the "MyVintasoftImageCollectionApiController" and press the "Add" button
- Specify that MyVintasoftImageCollectionApiController class is derived from Vintasoft.Imaging.AspNetCore.ApiControllers.VintasoftImageCollectionApiController class
Here are source codes of MyVintasoftImageCollectionApiController class:
using Microsoft.AspNetCore.Hosting;
namespace WebApplication1.Controllers
{
public class MyVintasoftImageCollectionApiController : Vintasoft.Imaging.AspNetCore.ApiControllers.VintasoftImageCollectionApiController
{
public MyVintasoftImageCollectionApiController(IWebHostEnvironment hostingEnvironment)
: base(hostingEnvironment)
{
}
}
}
-
Create web service that allows to get information about images, get thumbnails and render image tiles
- Press the right mouse button on the "Controllers" folder and select the "Add => Controller..." menu from context menu
- Select Empty API controller template, set the controller name to the "MyVintasoftImageApiController" and press the "Add" button
- Specify that MyVintasoftImageApiController class is derived from Vintasoft.Imaging.AspNetCore.ApiControllers.VintasoftImageApiController class
Here are source codes of MyVintasoftImageApiController class:
using Microsoft.AspNetCore.Hosting;
namespace WebApplication1.Controllers
{
public class MyVintasoftImageApiController : Vintasoft.Imaging.AspNetCore.ApiControllers.VintasoftImageApiController
{
public MyVintasoftImageApiController(IWebHostEnvironment hostingEnvironment)
: base(hostingEnvironment)
{
}
}
}
Compile the project for restoring dependencies using 'npm'.
-
Client side: Delete files, which are not necessary in this demo.
- Delete files "ClientApp\src\components\Counter.js", "ClientApp\src\components\FetchData.js", "ClientApp\src\components\Home.js",
"ClientApp\src\components\NavMenu.js", "ClientApp\src\components\NavMenu.css" - these React components are not necessary in this demo.
- Delete files "WeatherForecast.cs" and "Controllers\WeatherForecastController.cs" - the WeatherForecast Web API controller is not necessary in this demo.
-
Open file "ClientApp\src\components\Layout.js" and delete lines "import { NavMenu } from './NavMenu';" and "<NavMenu />" -
this demo does not need navigation menu.
-
Open file "ClientApp\src\App.js" and delete code that uses the following React components:
Home, FetchData, Counter - these React components are not necessary
in this demo.
Here are source codes of "App.js" file after update:
import React, { Component } from 'react';
import { Route } from 'react-router';
import { Layout } from './components/Layout';
import './custom.css'
export default class App extends Component {
static displayName = App.name;
render () {
return (
<Layout>
</Layout>
);
}
}
-
Client side: Add JavaScript files to the ASP.NET Core Web application.
- Copy Vintasoft.Shared.js and Vintasoft.Imaging.js files
from "<InstallPath>\VintaSoft Imaging .NET v12.4\Bin\JavaScript\" folder into the "ClientApp\public\" folder.
- Add references to Vintasoft JavaScript files to the header of "ClientApp\public\index.html" file:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<meta name="theme-color" content="#000000">
<base href="%PUBLIC_URL%/" />
<!--
manifest.json provides metadata used when your web app is added to the
homescreen on Android. See https://developers.google.com/web/fundamentals/engage-and-retain/web-app-manifest/
-->
<link rel="manifest" href="%PUBLIC_URL%/manifest.json">
<link rel="shortcut icon" href="%PUBLIC_URL%/favicon.ico">
<script src="Vintasoft.Shared.js" type="text/javascript"></script>
<script src="Vintasoft.Imaging.js" type="text/javascript"></script>
<!--
Notice the use of %PUBLIC_URL% in the tags above.
It will be replaced with the URL of the `public` folder during the build.
Only files inside the `public` folder can be referenced from the HTML.
Unlike "/favicon.ico" or "favicon.ico", "%PUBLIC_URL%/favicon.ico" will
work correctly both with client-side routing and a non-root public URL.
Learn how to configure a non-root public URL by running `npm run build`.
-->
<title>VintaSoft Document Viewer (ASP.NET Core + React.js)</title>
</head>
<body>
<noscript>
You need to enable JavaScript to run this app.
</noscript>
<div id="root"></div>
<!--
This HTML file is a template.
If you open it directly in the browser, you will see an empty page.
You can add webfonts, meta tags, or analytics to this file.
The build step will place the bundled scripts into the <body> tag.
To begin the development, run `npm start` or `yarn start`.
To create a production bundle, use `npm run build` or `yarn build`.
-->
</body>
</html>
- Add CSS-style "imageViewerContainer", which defines width and height of image viewer container, to the "ClientApp\src\custom.css" file,
add CSS-style "thumbnailViewerContainer", which defines width and height of thumbnail viewer container, to the "ClientApp\src\custom.css" file,:
/* Provide sufficient contrast against white background */
a {
color: #0366d6;
}
code {
color: #E01A76;
}
.btn-primary {
color: #fff;
background-color: #1b6ec2;
border-color: #1861ac;
}
.thumbnailViewerContainer {
width: 240px;
height: 650px;
float: left;
}
.imageViewerContainer {
width: 650px;
height: 650px;
float: left;
}
-
Client side: Create React.js component that displays image viewer.
- Create folder "wwwroot\UploadedImageFiles\SessionID" and copy test PDF document
"<SdkInstallPath>\VintaSoft\Imaging .NET v12.4\Images\VintasoftImagingDemo.pdf" to the folder.
This document will be displayed in document viewer.
- Create "ImageViewerDemo.js" file that will contain source codes of React.js component (ImageViewerDemo class):
- Select "Add => New Item..." context menu for folder "ClientApp\src\components\" => "Add new item" dialog will appear
- Select "JavaScript File" type for new item
- Set "ImageViewerDemo.js" as element name
- Click the "Add" button => dialog will be closed and file "ImageViewerDemo.js" will be added into folder "ClientApp\src\components\"
Add ImageViewerDemo class declaration with 'render' function (renders demo header and div element that will display image viewer) to the "ImageViewerDemo.js" file:
Add 'componentDidMount' function (contains JavaScript code that initializes and created image viewer) to the ImageViewerDemo class:
Here is JavaScript code of React.js component (ImageViewerDemo class):
import React, { Component } from 'react';
export class ImageViewerDemo extends Component {
render() {
return (
<div>
<h1>VintaSoft Image Viewer Demo (ASP.NET Core + React.js)</h1>
<div id="WebThumbnailViewer1Div" class="thumbnailViewerContainer"></div>
<div id="WebImageViewer1Div" class="imageViewerContainer"></div>
</div>
);
}
componentDidMount() {
// declare reference to the Vintasoft namespace
let Vintasoft = window.Vintasoft;
// set the session identifier
Vintasoft.Shared.WebImagingEnviromentJS.set_SessionId("SessionID");
// specify web services, which should be used by Vintasoft Web Document Viewer
Vintasoft.Shared.WebServiceJS.defaultFileService =
new Vintasoft.Shared.WebServiceControllerJS("vintasoft/api/MyVintasoftFileApi");
Vintasoft.Shared.WebServiceJS.defaultImageCollectionService =
new Vintasoft.Shared.WebServiceControllerJS("vintasoft/api/MyVintasoftImageCollectionApi");
Vintasoft.Shared.WebServiceJS.defaultImageService =
new Vintasoft.Shared.WebServiceControllerJS("vintasoft/api/MyVintasoftImageApi");
// create thumbnail viewer
let thumbnailViewer = new Vintasoft.Imaging.UI.WebThumbnailViewerJS("WebThumbnailViewer1Div");
// create image viewer
let imageViewer = new Vintasoft.Imaging.UI.WebImageViewerJS("WebImageViewer1Div");
// specify that image viewer depends from thumbnail viewer
imageViewer.set_MasterViewer(thumbnailViewer);
// open file from session folder and add images from file to the image viewer
imageViewer.get_Images().openFile("VintasoftImagingDemo.pdf");
}
}
- Add created React.js component to the React.js application code - "ClientApp\src\App.js" file.
Here are source codes of "App.js" file after update:
import React, { Component } from 'react';
import { Route } from 'react-router';
import { Layout } from './components/Layout';
import { ImageViewerDemo } from './components/ImageViewerDemo';
import './custom.css'
export default class App extends Component {
static displayName = App.name;
render () {
return (
<Layout>
<Route exact path='/' component={ImageViewerDemo} />
</Layout>
);
}
}
-
Run the ASP.NET Core Web application with React.js and see the result.